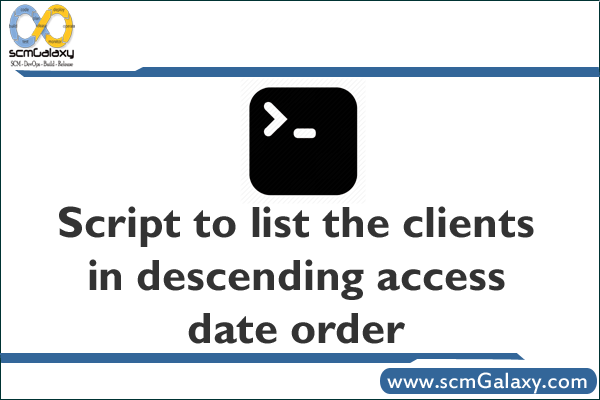
Write a script to list the clients in descending access date order (for deleting obsolete clients).
#!/usr/bin/ruby
require “P4”
p4 = P4.new
p4.tagged
p4.connect
clients = p4.run_clients.sort {|a,b| a[ “Access”].to_i <=> b[“Access”].to_i }
clients[0…10].each do
|c|
stamp = Time.at( c[ “Access” ].to_i )
printf( “%-20s %s\n”, c[ “client” ], stamp )
end
OR
#!/usr/bin/perl
use P4;
my $p4 = new P4;
$p4->Tag();
$p4->Init() or die( “Failed to connect to Perforce” );
my @clients = $p4->Clients();
@clients = sort { $a->{ “Access” } <=> $b->{ “Access” } } @clients;
@clients = @clients[ 0..9 ];
foreach my $client ( @clients )
{
last unless defined( $client );
my $stamp = localtime( $client->{ “Access” } );
printf( “%-20s %s\n”, $client->{ “client” }, $stamp );
}
- Installing Jupyter: Get up and running on your computer - November 2, 2024
- An Introduction of SymOps by SymOps.com - October 30, 2024
- Introduction to System Operations (SymOps) - October 30, 2024