How to check the given number is either Palindrome number or not in PHP?
What is the Palindrome number?
A palindrome is a word , number, phrase, verse or sentence that reads the same forward and backward.
Here , we’ll see the palindrome number:
Logic: Its logic has same like reverse number, in further we’ll check the given number and reverse number is equal or not.
- First of all, the remainder of $num divided by 10 is stored in the variable $rem. Now, $rem contains the last digit of $num, i.e. 1.
- $rem is then added to the variable $rev after multiplying it by 10.
- Multiplication by 10 adds a new place in the $rev contains. $rev contain like this 0 * 10 + 1 = 1.
- $num is then divided by 10 so that now it only contains first four digits: 1232.
- After second iteration, $rem equals 2, $rev equals 1 * 10 + 2 = 12 and $num = 123.
- After third iteration, $rem equals 3, $rev equals 12 * 10 + 3 = 123 and $num = 12.
- After fourth iteration, $rem equals 2, $rev equals 123 * 10 + 2 = 1232 and $num = 1.
- After fifth iteration, $rem equals 1, $rev equals 1232 * 10 + 1 = 12321 and $num = 0.
- Now, $num will be existed outside the while loop and $rev contains 12321.
Now , look at this coding
The input number is: 12321
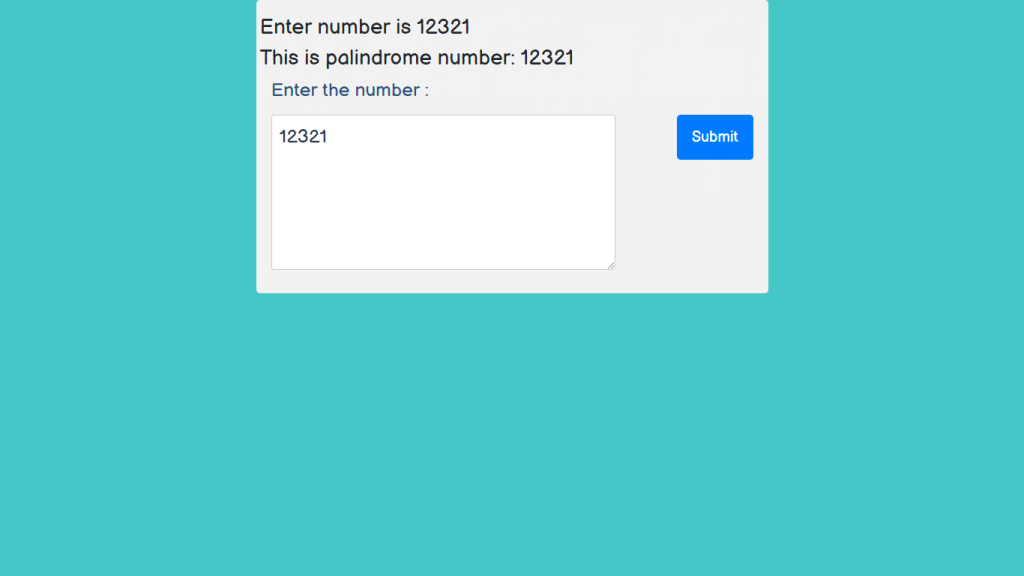
Another input is 1234:
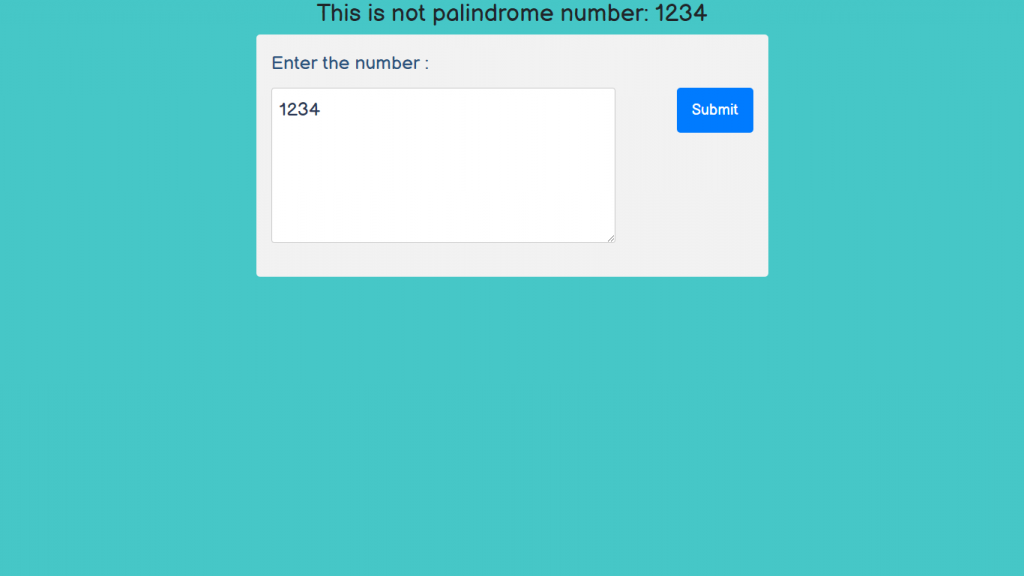
Thanks
Latest posts by Usha Kiran (see all)
- How to Login with Token in Laravel PHP Framework? - October 30, 2021
- How to merge two or multiple tables to each other in the Laravel PHP Framework? (Part-4) - October 29, 2021
- How to display a table in a Verticle or Horizontal form in the Laravel PHP Framework? Part-2 - October 29, 2021