How to Create a Simple Login Authentication System in LARAVEL?
Firstly, we’ll create a new project for this.
Step 1. Go to XAMPP/htdocs folder -> right Click->Open Git Bash Here


Step 2. To Create a New Project in Laravel, write down the following command:
$ composer create-project --prefer-dist laravel/laravel CustomerService "5.8.*"

Step 3. To Go the current working Project directory, write down this following command:
$ cd CustomerService
It’ll be redirected to the current Project.

Mysql Database connection Laravel
Step 4. To Create a new Database so we have to Go to XAMPP server->phpMyAdmin->Click New Database-> customerservice.

Step 5. Thereafter, Open Sublime Text and Go to .env file to set the current project path and give the project APP_URL path, DB_DATABASE name and DB_USERNAME name.


Step 6. To Migrate the user table which is in-built in database/migrations/ to Mysql database. Write down the following command:
$ php artisan migrate

Step 7. To Insert user records in users table by use of Laravel seeders. Write down the following command:
$ php artisan make:seeder UsersTablesSeeder

Step 8. Now, Go to the database/seeds/UsersTablesSeeder.php and define single user data in the run() method.
User::create([
'name' => 'Usha Kiran',
'email' => 'usha.cotocus@gmail.com',
'password' => Hash::make('password'),
'remember_token' => str_random(10),
]);

Step 9. After that, Go to the database/seeds/DatabaseSeeder.php and call the UsersTablesSeeder.php class within the run() method.

Step 10. Here, we’ll Insert data into the UsersTablesSeeders. Write down the following command:
$ php artisan db:seeder

Inserted date into users table

Step 11. To Create a Controller in the app/Http/Controllers folder. Write down the following command:
$ php artisan make:controller MainController --resource

Step 12. After that, we need to load the login form, write this code within the index() method.
public function index()
{
return view('login');
}

Step 13. So, Create login.blade.php file in resources/views to display

Step 14. Thereafter, Go to routes/web.php file and we’ll set route request.
Route::get('/login', 'MainController@index');


Thanks

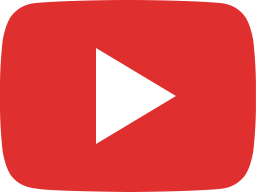

- How to Login with Token in Laravel PHP Framework? - October 30, 2021
- How to merge two or multiple tables to each other in the Laravel PHP Framework? (Part-4) - October 29, 2021
- How to display a table in a Verticle or Horizontal form in the Laravel PHP Framework? Part-2 - October 29, 2021