
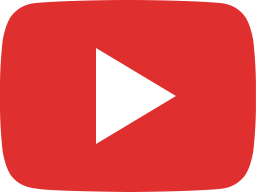
Laravel 5.5 CRUD Tutorial, Introduction And Agenda Overview, Laravel Training Part – 1 DevopsSchool

Latest posts by Deepak Kumar (see all)
- NPM Error: Node Sass does not yet support your current environment - November 10, 2020
- ERROR: sh: 1: cross-env: Permission denied - November 5, 2020
- Vue packages version mismatch laravel Error - September 15, 2020