How to merge two or multiple tables to each other in the Laravel PHP Framework?
How to seed Country/State data into the Database? Click Here Here, we are going to merge the Country table to the State table using left join
The LEFT JOIN keyword returns all records from the left table (table1), and the matched records from the right table (table2). The result is NULL from the right side if there is no match.
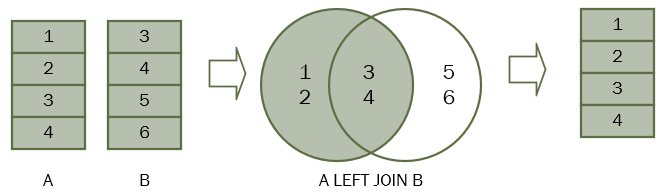
Step 1. Create a new Project in Laravel, so open git bash. Write down the following command:-
$ composer create-project --prefer-dist laravel/laravel Join "5.8.*"
Step 2. Create the authentication scaffolding and Country model. Write down the following command:-
$ php artisan make:auth
$ php artisan make:model Country -m
Step 3. Create the State model. Write down the following command:-
$ php artisan make:model State -m
Step 4. Generate a migration file into the database/migrations folder of the Country table.
public function up()
{
Schema::create('countries', function (Blueprint $table) {
$table->bigIncrements('country_id');
$table->string('country_name');
$table->string('sort');
$table->integer('phoneCode');
$table->timestamps();
});
}
Step 5. Generate a migration file into the database/migrations folder of State table.
public function up()
{
Schema::create('states', function (Blueprint $table) {
$table->bigIncrements('state_id');
$table->string('state_name');
$table->integer('country_id');
$table->timestamps();
});
}
Step 6. Migrate these tables into the MySQL database, so write the following command.
$ php artisan migrate
Step 7. Create a CountryController in App/Http/Controller folder so, write the following command.
$ php artisan make:controller CountryController --resource
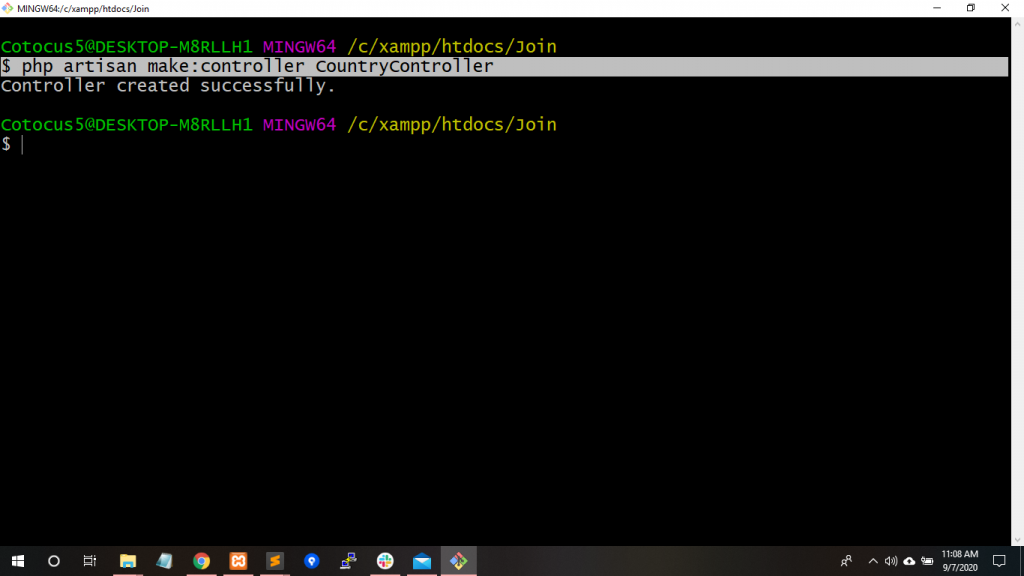
Step 8. Create a StateController in App/Http/Controller folder so, write the following command.
$ php artisan make:controller StateController --resource
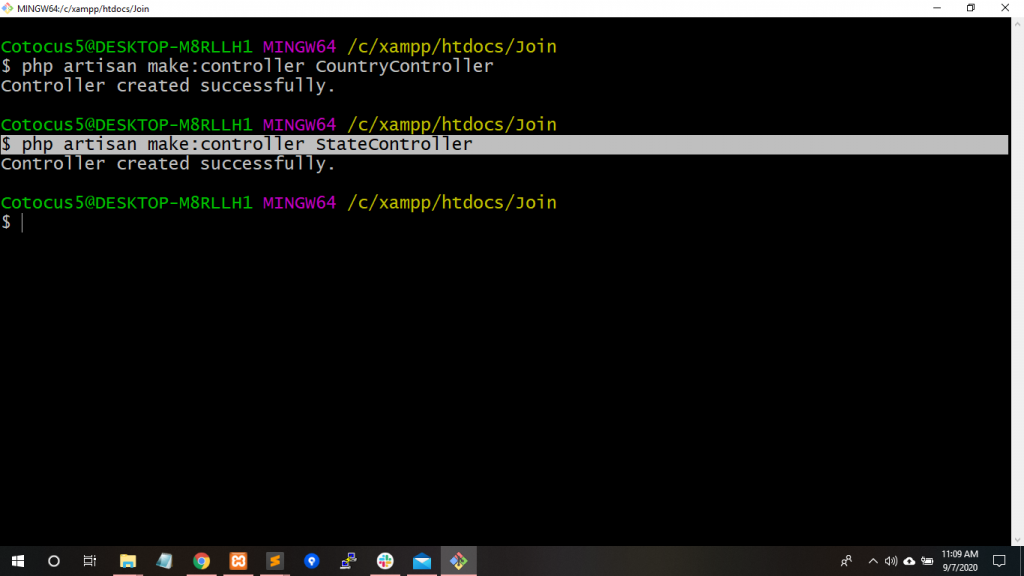
Step 9. Create child file resources/views/Country folder with name create.blade.php.
Step 10. Create an index.blade.php file within resource/views/Country/ folder.
Step 11. Create edit.blade.php file. In this file within resources/views/Country folder.
Thanks

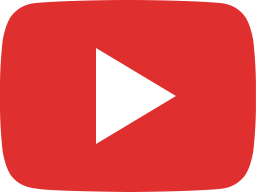

- How to Login with Token in Laravel PHP Framework? - October 30, 2021
- How to merge two or multiple tables to each other in the Laravel PHP Framework? (Part-4) - October 29, 2021
- How to display a table in a Verticle or Horizontal form in the Laravel PHP Framework? Part-2 - October 29, 2021