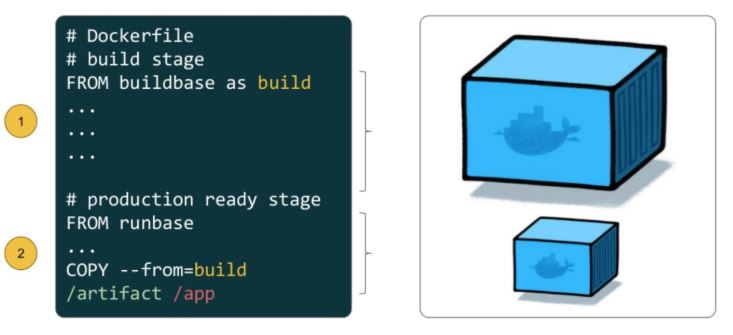
Problem with traditional approach of creating docker image?
One of the most challenging things about building images is keeping the image size down. Each instruction in the Dockerfile adds a layer to the image, and you need to remember to clean up any artifacts you don’t need before moving on to the next layer.
It was actually very common to have one Dockerfile to use for development (which contained everything needed to build your application), and a slimmed-down one to use for production, which only contained your application and exactly what was needed to run it. This has been referred to as the “builder pattern”. Maintaining two Dockerfiles is not ideal.
What is Docker multistaging build?
Multi-stage builds are a method of organizing a Dockerfile to minimize the size of the final container, improve run time performance, allow for better organization of Docker commands and files, and provide a standardized method of running build actions. A multi-stage build is done by creating different sections of a Dockerfile, each referencing a different base image. This allows a multi-stage build to fulfill a function previously filled by using multiple docker files, copying files between containers, or running different pipelines.
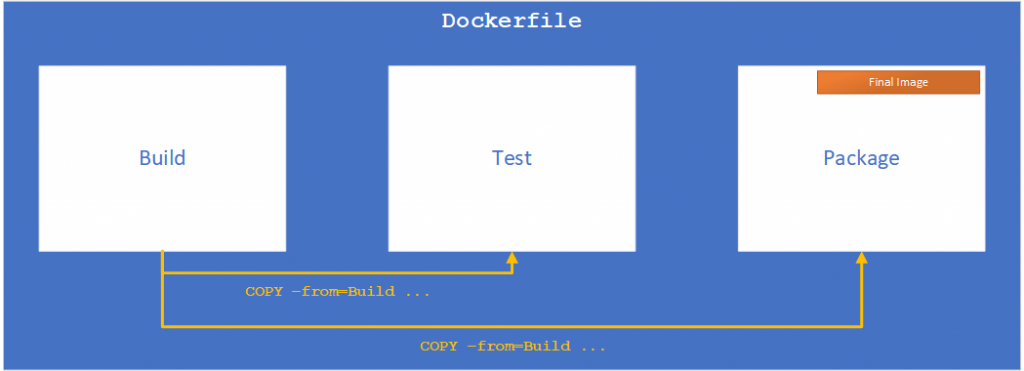
Before multi-stage builds
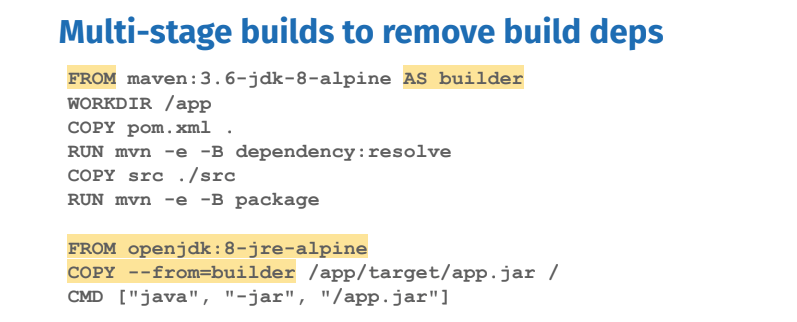
After Use multi-stage builds
You only need the single Dockerfile. You don’t need a separate build script, either. Just run docker build.
Docker Tutorials Fundamental To Advanced-2021 Crash Course:- https://bit.ly/3hOIbTB

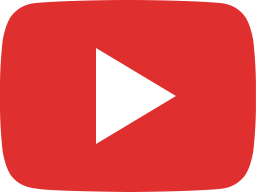
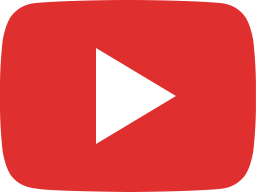
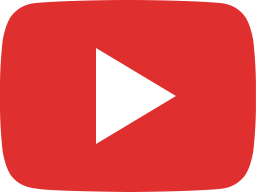
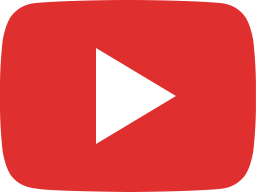
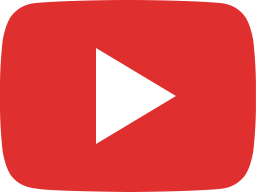
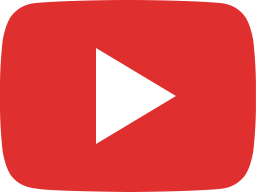

- Best Hospitals for affordable surgery for medical tourism - December 20, 2024
- Top Global Medical Tourism Companies in the World - December 20, 2024
- Medical Laboratory equipment manufacturing company - December 20, 2024