How to Store Countries, States, Cities seed classes into the Database in Laravel PHP?
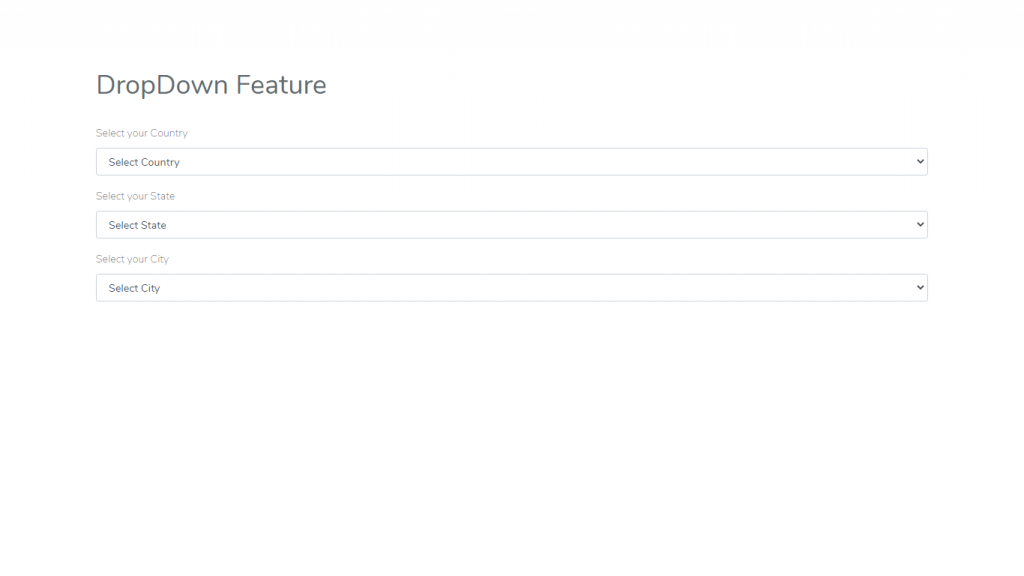
Step 1. Create a Country seeder file. Write down the following command as follows:
$ php artisan make:seeder CountriesTableSeeder
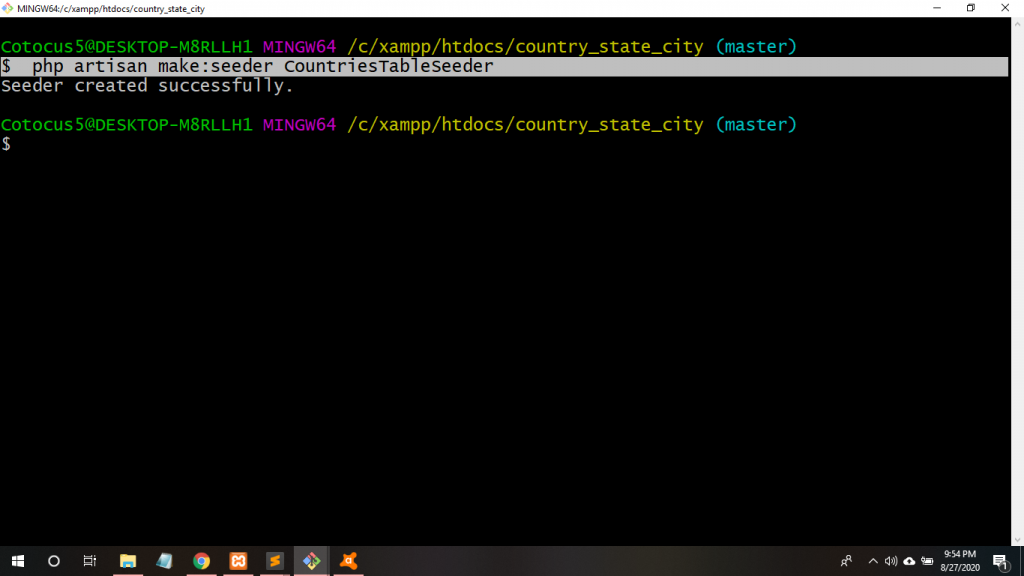
Step 2. Create a Country model. Write down the following command as follows:
$ php artisan make:model Country -m
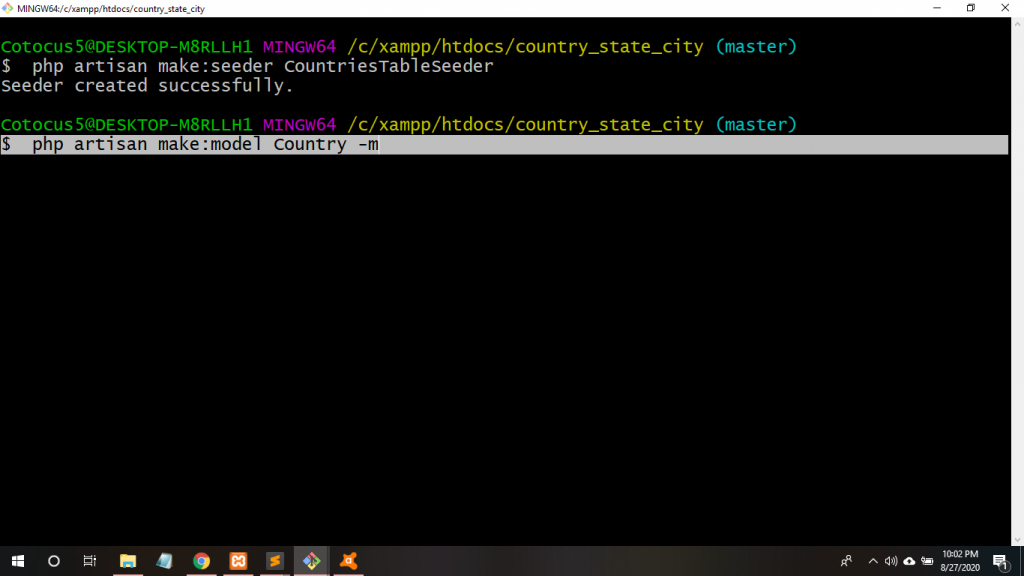
Step 3. Add columns into Country table.
public function up()
{
Schema::create('countries', function (Blueprint $table) {
$table->bigIncrements('id');
$table->string('sort');
$table->string('country_name');
$table->integer('phoneCode');
$table->timestamps();
});
}
Step 4. Now, Go to Database/seeds/CountriesTableSeeder file.
Step 5. After that Go to Database/seeds/DatabaseSeeder file. Write down the following command as follows:
public function run()
{
$this->call(CountriesTableSeeder::class);
}
Step 6. Migrate the tables into the MySQL database.
$ php artisan migrate
Step 7. Run the seeder class file individually.
$ php artisan db:seed --class=CountriesTableSeeder
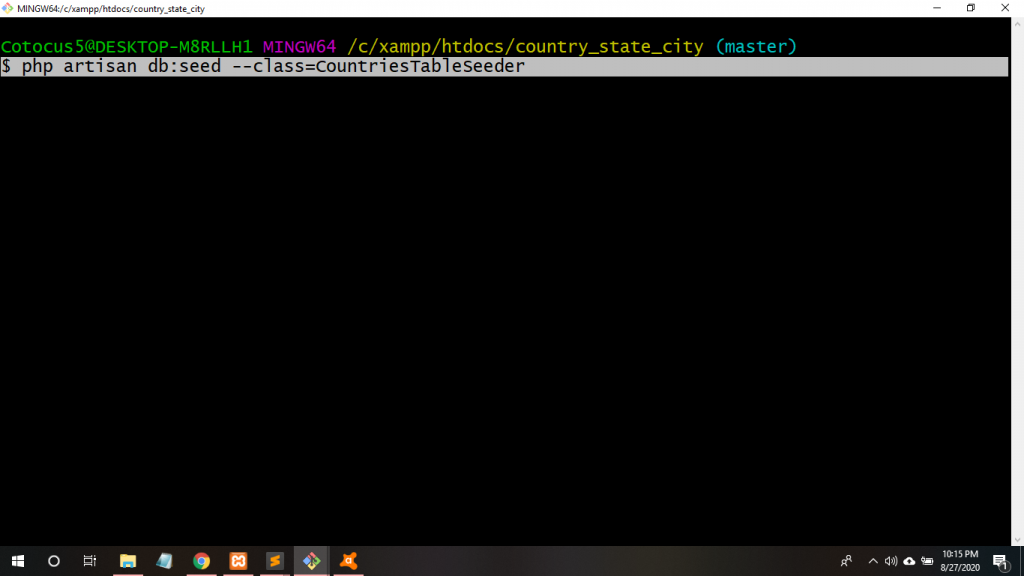
Step 8. Go to Database/seeds/CountriesTableSeeder.php file
Step 9. Then, go to routes/web.php file and define all these routes.
Route::get('/','MainController@index');
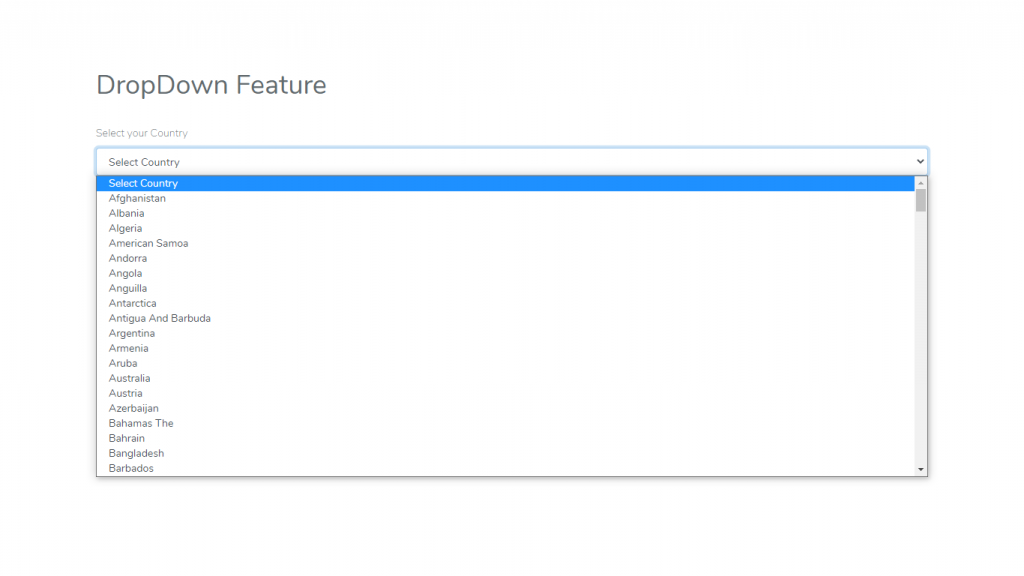
Thanks

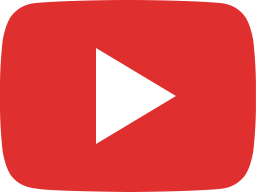

- How to Login with Token in Laravel PHP Framework? - October 30, 2021
- How to merge two or multiple tables to each other in the Laravel PHP Framework? (Part-4) - October 29, 2021
- How to display a table in a Verticle or Horizontal form in the Laravel PHP Framework? Part-2 - October 29, 2021