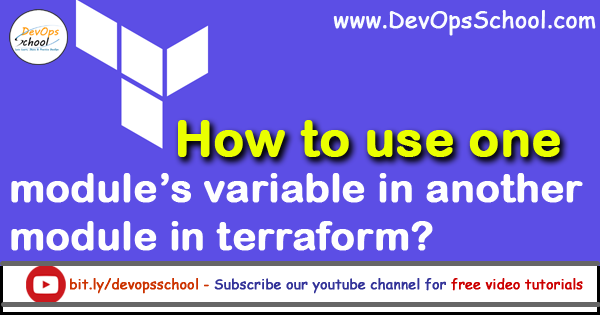
To get the output from one module to another module in Terraform, you can use the following steps:
- Define the output in the first module.
- Import the second module.
- Reference the output of the first module in the second module.
Here is an example of how to do this:
# First module
module "web_server" {
source = "./web_server"
output "instance_ip_addr" {
value = aws_instance.web_server.public_ip
}
}
# Second module
module "database" {
source = "./database"
instance_ip_addr = module.web_server.instance_ip_addr
}
In this example, the web_server
module defines an output called instance_ip_addr
. The database
module imports the web_server
module and then references the instance_ip_addr
output in its own configuration.
When you run terraform apply
, Terraform will create the resources defined in both modules. The database
module will use the instance_ip_addr
output from the web_server
module to connect to the web server.
Here is an explanation of the code:
- The
source
attribute in themodule
block specifies the location of the module definition. In this case, the module definition is located in theweb_server
directory. - The
output
block defines an output calledinstance_ip_addr
. The value of the output is the public IP address of the web server instance. - The
source
attribute in themodule
block specifies the location of the module definition. In this case, the module definition is located in thedatabase
directory. - The
instance_ip_addr
attribute references theinstance_ip_addr
output from theweb_server
module.
You can use outputs to accomplish this. In Terraform, a module can also return values. Again, this is done using a mechanism you already know: output variables. Such as if there are 2 modules, kafka and cloudwatch.
In your kafka module, you could define an output that looks something like this:
output "instance_ids" {
value = ["${aws_instance.kafka.*.id}"]
}
Case 1 – If you are calling kafka module from cloudwatch module by instantiated the module with something like:
module "kafka" {
source = "./modules/kafka"
}
# You can then access that output as follows:
instances = ["${module.kafka.instance_ids}"]
Case 2 – If your modules are isolated from each other (i.e. your cloudwatch module doesn’t instantiate your kafka module), you can pass the outputs as variables between modules:
module "kafka" {
source = "./modules/kafka"
}
module "cloudwatch" {
source = "./modules/cloudwatch"
instances = ["${module.kafka.instance_ids}"]
}
# Of course, your "cloudwatch" module would have to declare the instances variable.
Case 3 – You can add the ASG name as an output variable in /modules/services/webserver-cluster/outputs.tf as follows.
output "asg_name" {
value = aws_autoscaling_group.example.name
description = "The name of the Auto Scaling Group"
}
You can access module output variables the same way as resource output attributes. The syntax is:
module.<MODULE_NAME>.<OUTPUT_NAME>
module.frontend.asg_name

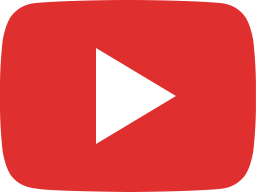
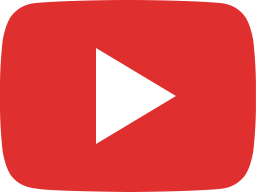
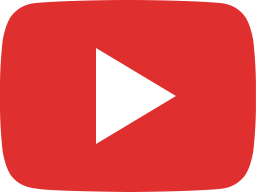
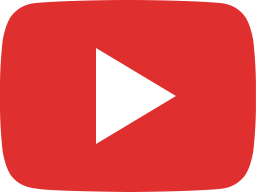
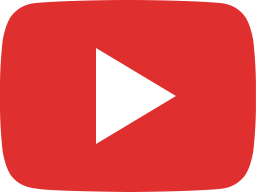
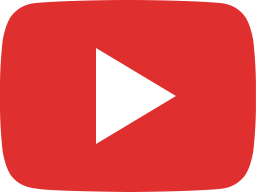
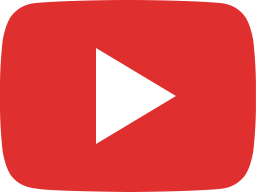
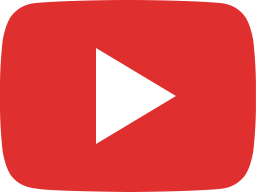

I’m a DevOps/SRE/DevSecOps/Cloud Expert passionate about sharing knowledge and experiences. I am working at Cotocus. I blog tech insights at DevOps School, travel stories at Holiday Landmark, stock market tips at Stocks Mantra, health and fitness guidance at My Medic Plus, product reviews at I reviewed , and SEO strategies at Wizbrand.
Please find my social handles as below;
Rajesh Kumar Personal Website
Rajesh Kumar at YOUTUBE
Rajesh Kumar at INSTAGRAM
Rajesh Kumar at X
Rajesh Kumar at FACEBOOK
Rajesh Kumar at LINKEDIN
Rajesh Kumar at PINTEREST
Rajesh Kumar at QUORA
Rajesh Kumar at WIZBRAND