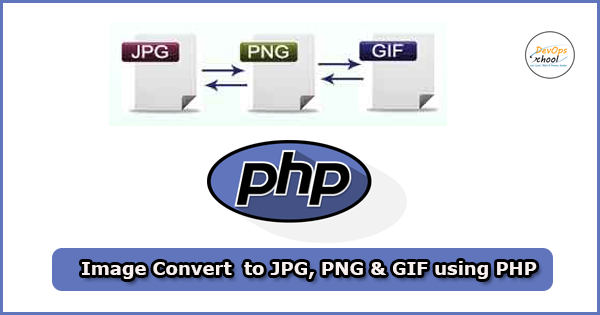
In this post I help you to convert any uploaded image to JPG, PNG and GIF.
Create a file image_converter.php.
In this code you see using convert_image() has three mandatory parameters like as:
$convert_type => accepts string either png,jpg or gif.
$target_dir => it is the source as well as the target directory
$image_name => give the actual image name such as image1.jpg.
$image_quality => can be adjusted, if you don’t want 100% quality.
Next, create index.php to Upload the image.
Then, create convert.php to convert the image. And make a folder in your directory name as uploads. Because when the image is uploaded or convert, the image will save on this folder.
And, create a download.php to downloads the converted image forcefully.
Then, Run the index.php and see the view.
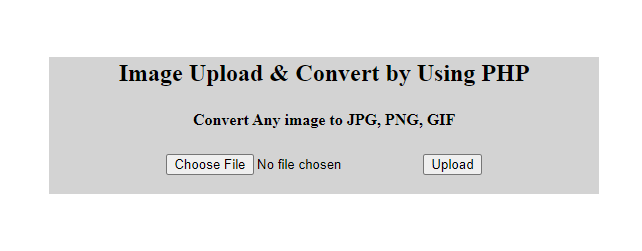
After Upload the image.
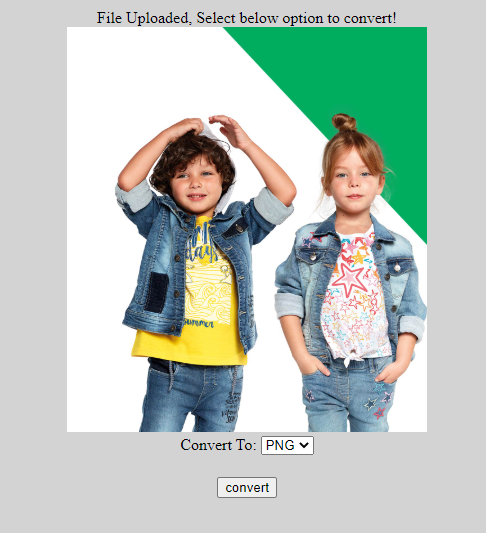
After converted the image.
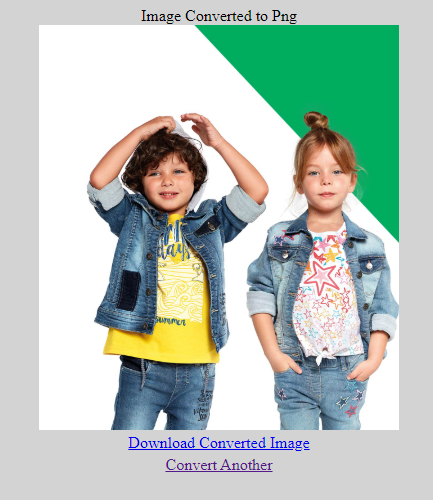
Then, you can download or convert another image.

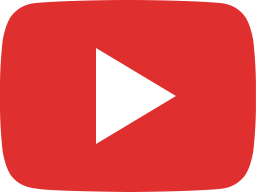
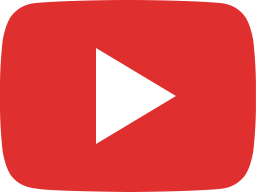

- Top 5 Reliable Phone Number Validation API for Your Next Product - February 10, 2022
- Top 5 IT Management Tools | IT Management Software - July 30, 2021
- Complete guide of PHP certification courses, tutorials & training - July 23, 2021