Introduction:
Hello Dear Reader,
In this post, I am going to demonstrate detailed steps of how to send email to user’s email id with the files that were submitted by the user as an attachment
The complete working example code has been given in the code section
below
Create all the files and then run command npm run dev
in your project directory before testing
Also, the explanation has been provided in the bottom section of the post
Enjoy!
Explanation:
Step 1:Enable JQuery in your Laravel application
Enable JQuery in your Laravel application by adding below line of code in your blade file or the parent blade file:<script src="{{ asset('js/app.js') }}"></script>
Refer Laravel-Email-Project\resources\views\layouts\app.blade.php
NOTE: How you make sure, adding above line of code will enable JQuery?
ANS: When you open resources\assets\js\app.js file, you will see it requires ./bootsrap.js file. Now open bootstrap.js file, you will see it has a require statement for importing JQuery. The line in bootstrap.js will look something like shown below:try {
window.$ = window.jQuery = require('jquery');
//require('bootstrap-sass'); require('bootstrap'); } catch (e) {}
Hence, the above code explains that app.js imports JQuery from the node modules folder and makes it available for all the blade file that uses app.js.
Step 2: Create a form Laravel-Email-Project\resources\views\pages\user-files-form.blade.php, that accepts one file or more than one files from the user. Make sure to have enctype="multipart/form-data"
in the form tag
Also, don’t forget to import Laravel-Email-Project\resources\assets\js\pages\user-files-script.js
Step 3: Create a Controller file that accepts the request with files: Laravel-Email-Project\app\Http\Controllers\UserFilesEmailController.php
Step 4: Create a route in web.php
Step 5: Create Laravel-Email-Project\app\Notifications\Mails\Users\UserFilesEmail.php, that will have code to form email and trigger the email with attachments
Step 6: Create Laravel-Email-Project\resources\assets\js\pages\user-files-script.js that will have jquery code to submit the form with files input
Step 7: Create Laravel-Email-Project\resources\views\notifications\mails\users\user-files-email-plain.blade.php that will have text version of the email template
Step 8: Create Laravel-Email-Project\resources\views\notifications\mails\users\user-files-email.blade.php that will have the template of the email body
Step 9: Add the compilation & migration of the user-files-script.js from assets to public directory in Laravel-Email-Project\webpack.mix.js file
Step 10: Open git bash in the project directory and run command: npm run dev

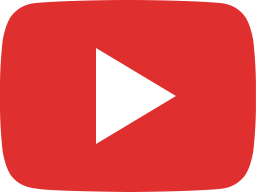
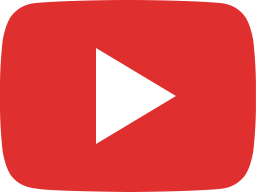

- MyHospitalNow.com – PROD | Clean all patient user and related quote, bid and file data - March 21, 2020
- Sending Email with attachments in Laravel 5.5 using and PHP & JQuery - February 20, 2020
- How to run php artisan queue:listen in background on Ubuntu or Linux machine - February 6, 2020