How to Create a Simple Login Authentication System in LARAVEL?
Firstly, Visit this Blog Part-1.
Step 15. Now add these classes within MainController file.
use Validator;
use Auth;
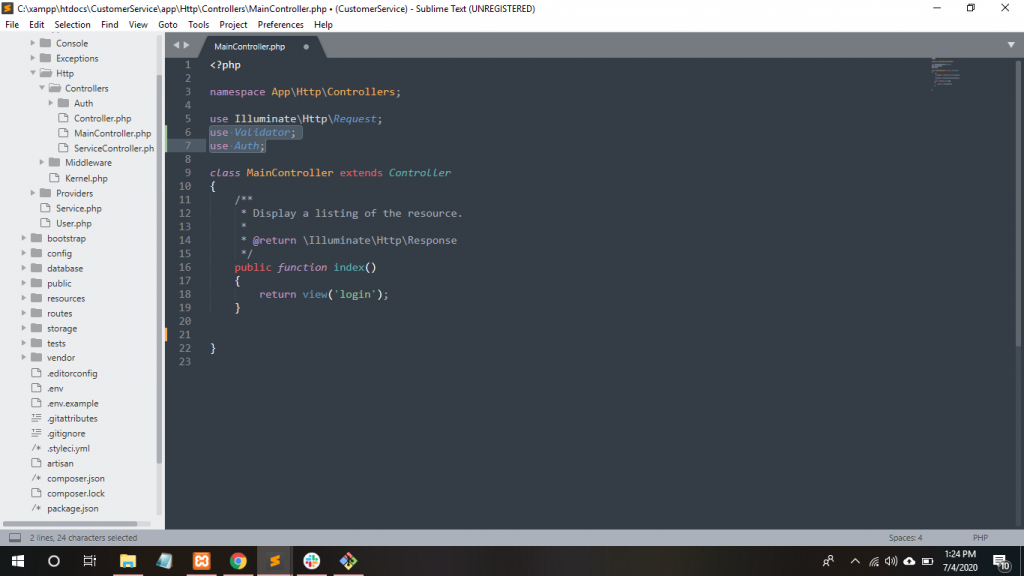
Step 16. Create a function checklogin to receive login form request within the MainController file.
function checklogin(Request $request)
{
$this->validate($request, [
'email' => 'required|email',
'password' => 'required|alphaNum|min:3'
]);
$user_data = array(
'email' => $request->get('email'),
'password' => $request->get('password')
);
if(Auth::attempt($user_data))
{
return redirect('main/successlogin');
}
else
{
return back()->with('error', 'Wrong Login Details');
}
}
Step 17. Write down the following code within the login form file for validation error:
@if (count($errors) > 0)
<div class="alert alert-danger">
<ul>
@foreach($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
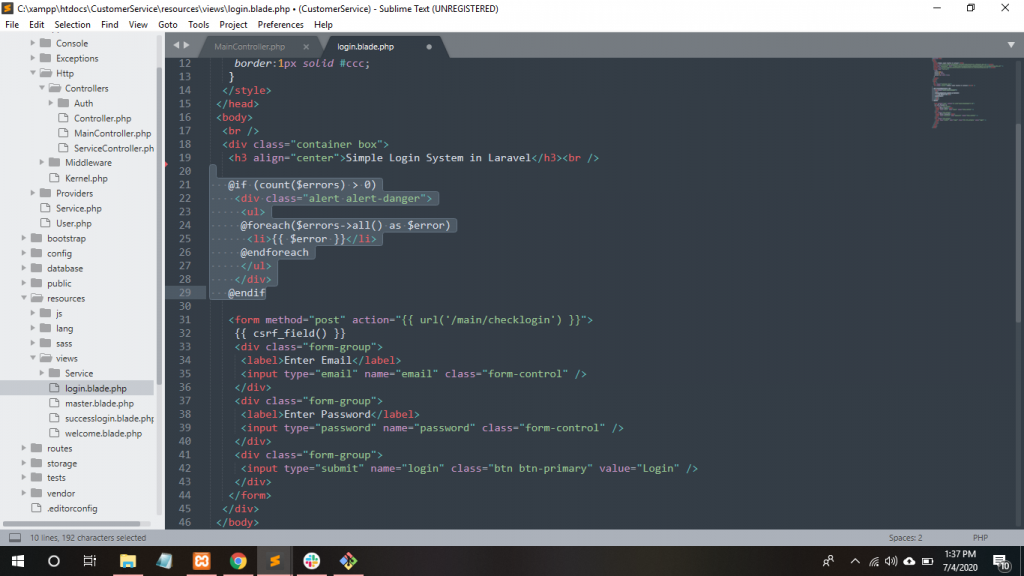
Step 18. Write down the following code to display an error message within login form file:
@if ($message = Session::get('error'))
<div class="alert alert-danger alert-block">
<button type="button" class="close" data-dismiss="alert">Γ</button>
<strong>{{ $message }}</strong>
</div>
@endif
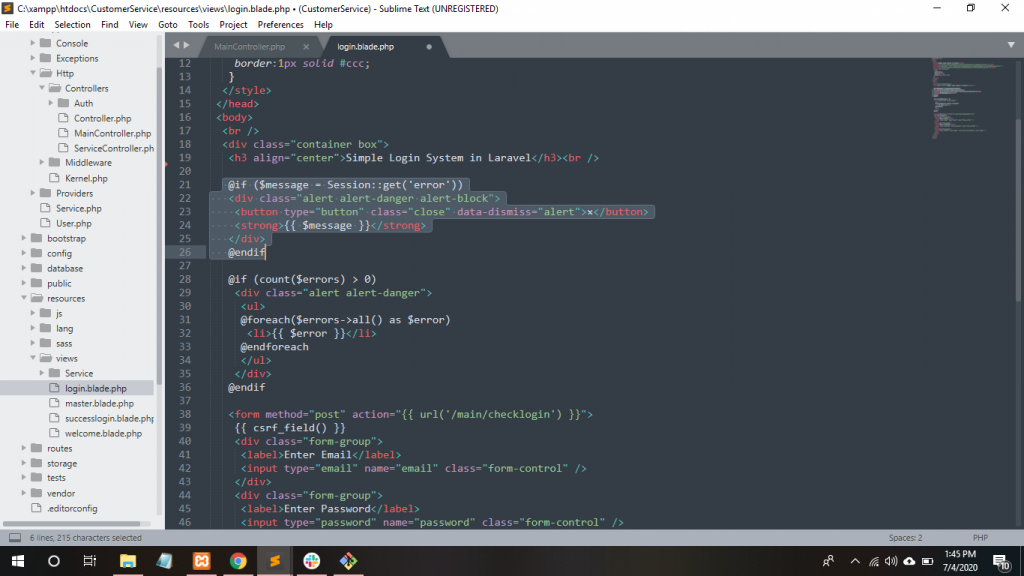
Step 19. Now, Create a function checklogin to within the MainController file.
function successlogin()
{
return view('successlogin');
}
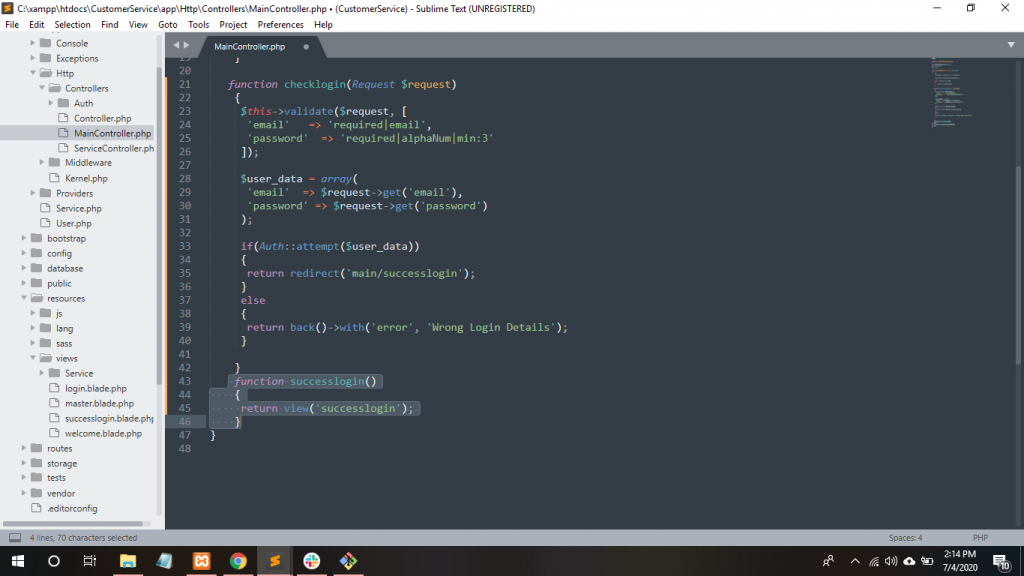
Step 20. Create a successlogin form file within resources/views
<!DOCTYPE html> | |
<html> | |
<head> | |
<title>Simple Login System in Laravel</title> | |
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script> | |
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" /> | |
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> | |
<style type="text/css"> | |
.box{ | |
width:600px; | |
margin:0 auto; | |
border:1px solid #ccc; | |
} | |
</style> | |
</head> | |
<body> | |
<br /> | |
<div class="container box"> | |
<h3 align="center">Simple Login System in Laravel</h3><br /> | |
@if(isset(Auth::user()->email)) | |
<div class="alert alert-danger success-block"> | |
<strong>Welcome {{ Auth::user()->email }}</strong> | |
<br /> | |
<a href="{{ url('/main/logout') }}">Logout</a> | |
</div> | |
@else | |
<script>window.location = "/main";</script> | |
@endif | |
<br /> | |
</div> | |
</body> | |
</html> |
Step 21. Create a function logout to within the MainController file.
function logout()
{
Auth::logout();
return redirect('main');
}
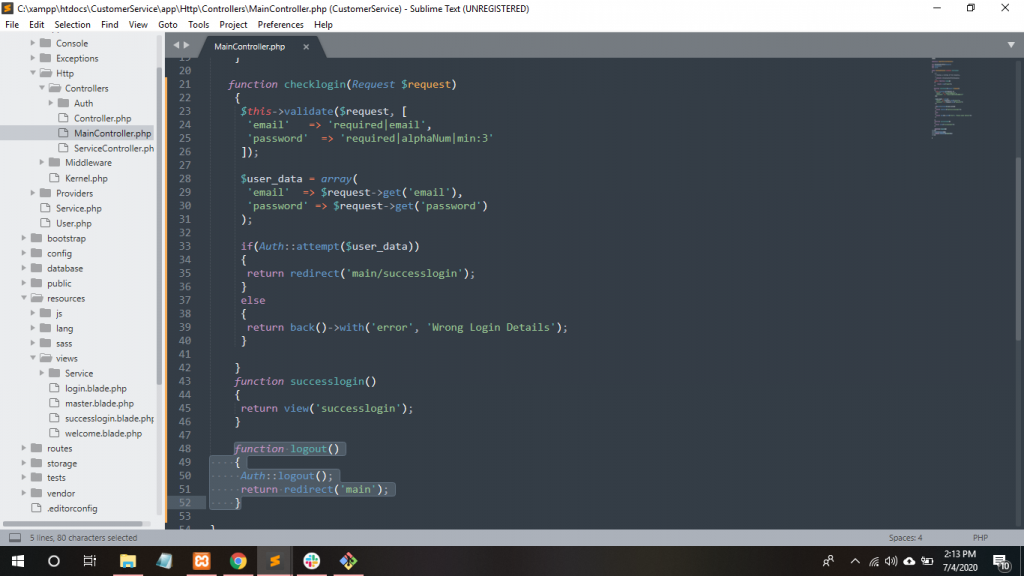
<?php | |
namespace App\Http\Controllers; | |
use Illuminate\Http\Request; | |
use Validator; | |
use Auth; | |
class MainController extends Controller | |
{ | |
/** | |
* Display a listing of the resource. | |
* | |
* @return \Illuminate\Http\Response | |
*/ | |
public function index() | |
{ | |
return view('login'); | |
} | |
function checklogin(Request $request) | |
{ | |
$this->validate($request, [ | |
'email' => 'required|email', | |
'password' => 'required|alphaNum|min:3' | |
]); | |
$user_data = array( | |
'email' => $request->get('email'), | |
'password' => $request->get('password') | |
); | |
if(Auth::attempt($user_data)) | |
{ | |
return redirect('main/successlogin'); | |
} | |
else | |
{ | |
return back()->with('error', 'Wrong Login Details'); | |
} | |
} | |
function successlogin() | |
{ | |
return view('successlogin'); | |
} | |
function logout() | |
{ | |
Auth::logout(); | |
return redirect('main'); | |
} | |
} |
Step 22. Go to web.php file and we have to set route of all requests.
Route::get('/main', 'MainController@index');
Route::post('/main/checklogin', 'MainController@checklogin');
Route::get('main/successlogin', 'MainController@successlogin');
Route::get('main/logout', 'MainController@logout');
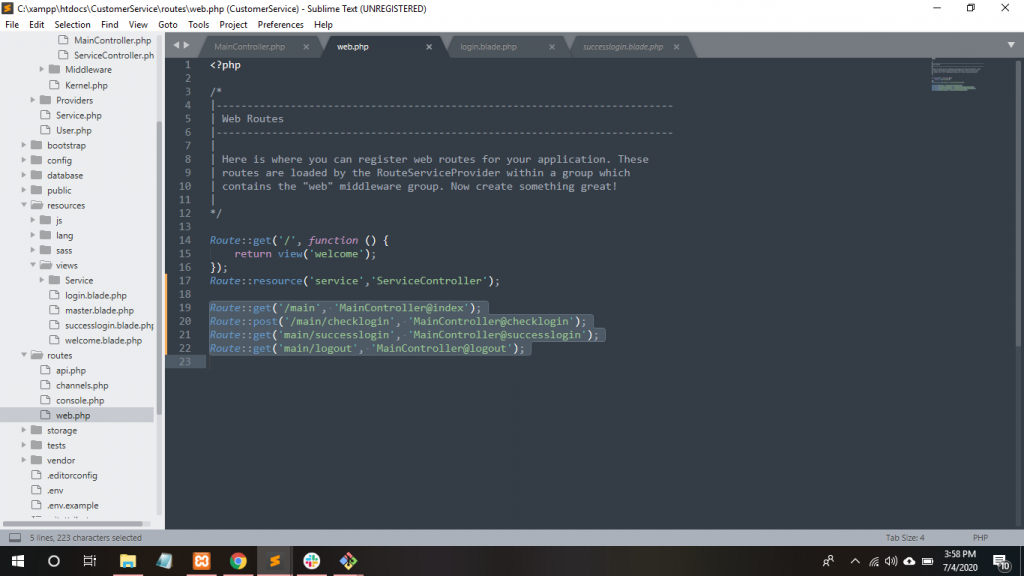
Step 23. Write down the following statement code within the login form.
@if(isset(Auth::user()->email))
<script>window.location="/main/successlogin";</script>
@endif
<!DOCTYPE html> | |
<html> | |
<head> | |
<title>Simple Login System in Laravel</title> | |
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script> | |
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" /> | |
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> | |
<style type="text/css"> | |
.box{ | |
width:600px; | |
margin:0 auto; | |
border:1px solid #ccc; | |
} | |
</style> | |
</head> | |
<body> | |
<br /> | |
<div class="container box"> | |
<h3 align="center">Simple Login System in Laravel</h3><br /> | |
@if(isset(Auth::user()->email)) | |
<script>window.location="/main/successlogin";</script> | |
@endif | |
@if ($message = Session::get('error')) | |
<div class="alert alert-danger alert-block"> | |
<button type="button" class="close" data-dismiss="alert">Γ</button> | |
<strong>{{ $message }}</strong> | |
</div> | |
@endif | |
@if (count($errors) > 0) | |
<div class="alert alert-danger"> | |
<ul> | |
@foreach($errors->all() as $error) | |
<li>{{ $error }}</li> | |
@endforeach | |
</ul> | |
</div> | |
@endif | |
<form method="post" action="{{ url('/main/checklogin') }}"> | |
{{ csrf_field() }} | |
<div class="form-group"> | |
<label>Enter Email</label> | |
<input type="email" name="email" class="form-control" /> | |
</div> | |
<div class="form-group"> | |
<label>Enter Password</label> | |
<input type="password" name="password" class="form-control" /> | |
</div> | |
<div class="form-group"> | |
<input type="submit" name="login" class="btn btn-primary" value="Login" /> | |
</div> | |
</form> | |
</div> | |
</body> | |
</html> |
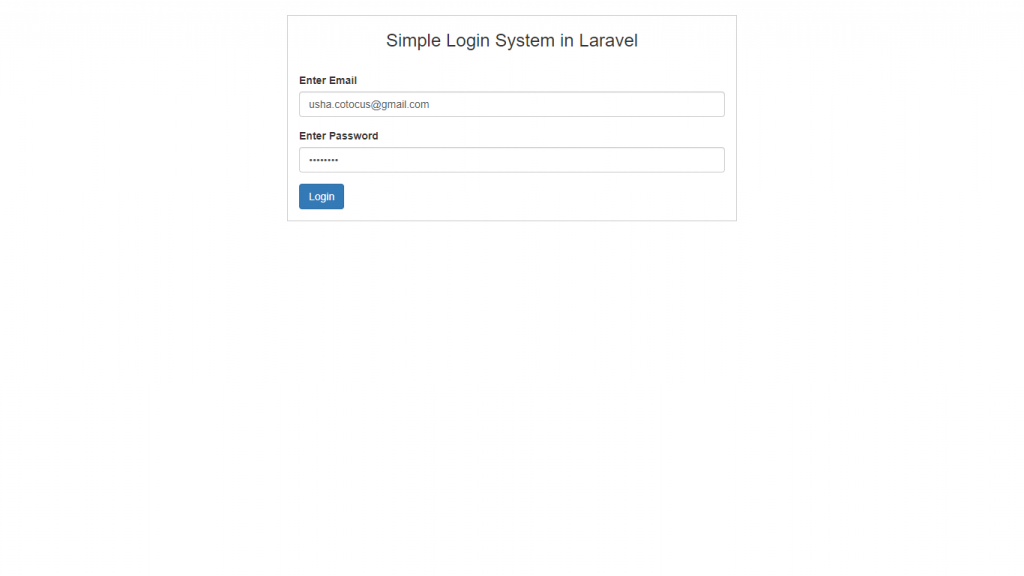
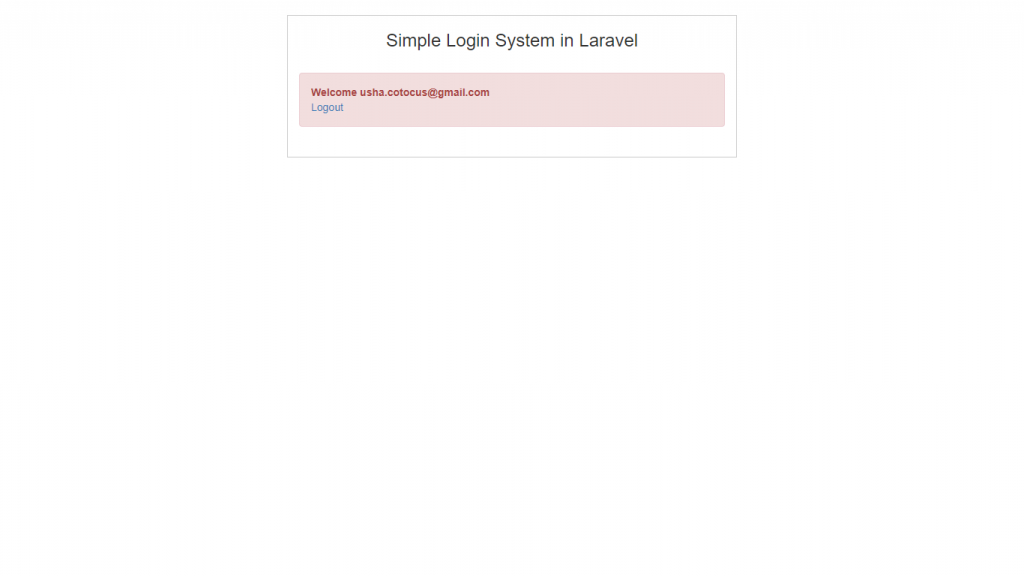
Thanks

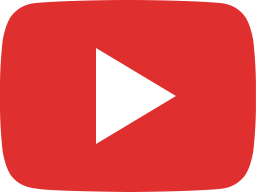

With MotoShare.in, you can book a bike instantly, enjoy doorstep delivery, and ride without worries. Perfect for travelers, professionals, and adventure enthusiasts looking for a seamless mobility solution.