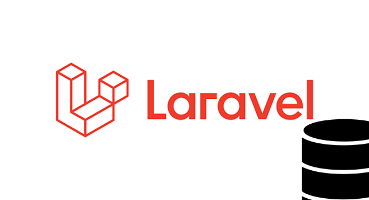
-
Laravel Migration Example Tutorial. This tutorial explain you step by step, how you can add single or multiple columns in your existing database tables.
-
Sometimes there is a need to add new columns in your database tables. Today we will show you how to add new columns or columns to an existing table using Laravel migration.
Add column name into the school.php model
.
To add a new column to the existing table using the laravel migration. Let’s open your terminal and create a migration file using the below command:
php artisan make:migration add_phone_number_to_schools
<?php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class AddPhoneNumberToSchools extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('schools', function (Blueprint $table) {
$table->string('phone_number', 10)->nullable(false)->default(null);
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('schools', function (Blueprint $table) {
$table->dropColumn('phone_number');
});
}
}
--Initially schools table in db looks like this:
After running below command phone_number
column will reflect.
php artisan migrate
--Now, schools table in db looks like this:
php artisan make:migration add_multiple_column_to_schools
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class School extends Model
{
protected $fillable = [
'school_name', 'email', 'country', 'phone_number', 'Afiliated_by', 'School_location', 'Medium'
];
}
<?php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class AddMultipleColumnToSchools extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('schools', function (Blueprint $table) {
$table->string('afiliated_by')->nullable();
$table->string('short_description')->nullable();
$table->string('medium')->nullable()
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('schools', function (Blueprint $table) {
$table->dropColumn(['afiliated_by', 'short_description', 'medium']);
});
}
}
** Check whether multiple Column has been added succesfully.. **

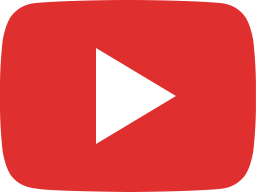

MotoShare.in is your go-to platform for adventure and exploration. Rent premium bikes for epic journeys or simple scooters for your daily errands—all with the MotoShare.in advantage of affordability and ease.