How to display a table in a Verticle or Horizontal form in the Laravel PHP Framework?
Step 1. Create an index.blade.php file within resource/views/Country/ folder.
Step 2. Create a Controller within the App/Http/Controller folderCountryController.php file. So, write the following command.
$ php artisan make:controller CountryController --resource
Step 3. Go to routes/web.php file ,we have to define route of this all CountryController CRUD functions.
Route::resource('country','CountryController');
Now Displaying Horizontal way
There are two headings that are shown on the horizontal, it’s displayed in the row format.
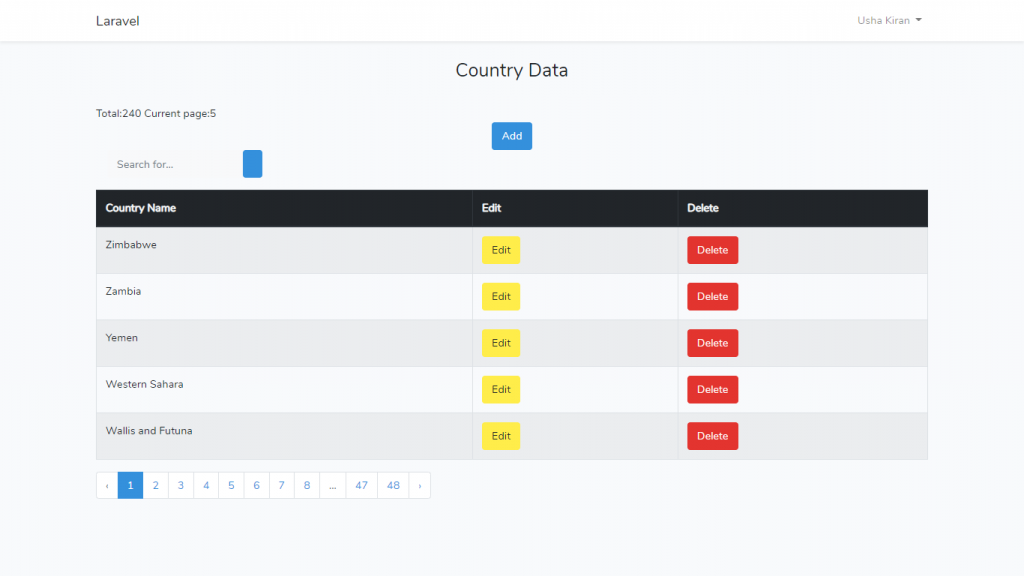
Step 1. Go to resources/views/country/index.blade.php file
Thanks

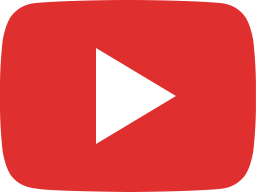
Laravel 5.5 CRUD Tutorial, Introduction And Agenda Overview, Laravel Training Part – 1 DevopsSchool

Latest posts by Usha Kiran (see all)
- How to Login with Token in Laravel PHP Framework? - October 30, 2021
- How to merge two or multiple tables to each other in the Laravel PHP Framework? (Part-4) - October 29, 2021
- How to display a table in a Verticle or Horizontal form in the Laravel PHP Framework? Part-2 - October 29, 2021