How to merge two or multiple tables to each other in the Laravel PHP Framework?
Step 1. Go to app/Country.php. Now, we need to list all the properties we need in the $fillable array.
class Country extends Model | |
{ | |
protected $fillable = ['country_name']; | |
} |
Step 2. Go to app/Http/Controllers/CountryController.php file.
<?php | |
namespace App\Http\Controllers; | |
use Illuminate\Http\Request; | |
use App\Http\Controllers\Controller; | |
use Illuminate\Support\Facades\Auth; | |
use Illuminate\Support\Facades\DB; | |
use Illuminate\Support\Facades\Input; | |
use App\Country; | |
class CountryController extends Controller | |
{ | |
/** | |
* Display a listing of the resource. | |
* | |
* @return \Illuminate\Http\Response | |
*/ | |
public function index() | |
{ | |
$countries = Country::all()->toArray(); | |
$countries = DB::table('countries')->orderBy('country_id','desc')->paginate(5); | |
return view('country.index', ['countries' => $countries]); | |
} | |
/** | |
* Show the form for creating a new resource. | |
* | |
* @return \Illuminate\Http\Response | |
*/ | |
public function create() | |
{ | |
return view('country.create'); | |
} | |
public function search(Request $request) | |
{ | |
$search = $request->get('search'); | |
if($search != ''){ | |
$countries = Country::where('country_name','like', '%' .$search. '%')->paginate(2); | |
$countries->appends(array('search'=> Input::get('search'),)); | |
if(count($countries )>0){ | |
return view('country.index',['countries'=>$countries]); | |
} | |
return back()->with('error','No results Found'); | |
} | |
} | |
public function store(Request $request) | |
{ | |
$this->validate($request, [ | |
'country_name' => 'required', | |
]); | |
$country = new Country([ | |
'country_name' => $request->get('country_name') | |
]); | |
$country->save(); | |
return redirect()->route('country.create')->with('success', 'Data Added'); | |
} | |
/** | |
* Display the specified resource. | |
* | |
* @param int $id | |
* @return \Illuminate\Http\Response | |
*/ | |
public function show($id) | |
{ | |
// | |
} | |
/** | |
* Show the form for editing the specified resource. | |
* | |
* @param int $id | |
* @return \Illuminate\Http\Response | |
*/ | |
public function edit($id) | |
{ | |
$country = Country::where('country_id',$id)->first(); | |
return view('country.edit', compact('country')); | |
} | |
/** | |
* Update the specified resource in storage. | |
* | |
* @param \Illuminate\Http\Request $request | |
* @param int $id | |
* @return \Illuminate\Http\Response | |
*/ | |
public function update(Request $request, $id) | |
{ | |
$this->validate($request, [ | |
'country_name' => 'required', | |
]); | |
$data = array( | |
'country_name' => $request->country_name | |
); | |
Country::where('country_id', $id)->update($data); | |
return redirect()->route('country.index')->with('success', 'Data Updated'); | |
} | |
/** | |
* Remove the specified resource from storage. | |
* | |
* @param int $id | |
* @return \Illuminate\Http\Response | |
*/ | |
public function destroy($id) | |
{ | |
$country = Country::where('country_id', $id); | |
$country->delete(); | |
return redirect()->route('country.index')->with('success', 'Data Deleted'); | |
} | |
} |
Step 3. Then, go to routes/web.php file and define all these routes.
Route::resource('country','CountryController');
Route::any('/search', 'CountryController@search');
Run URL
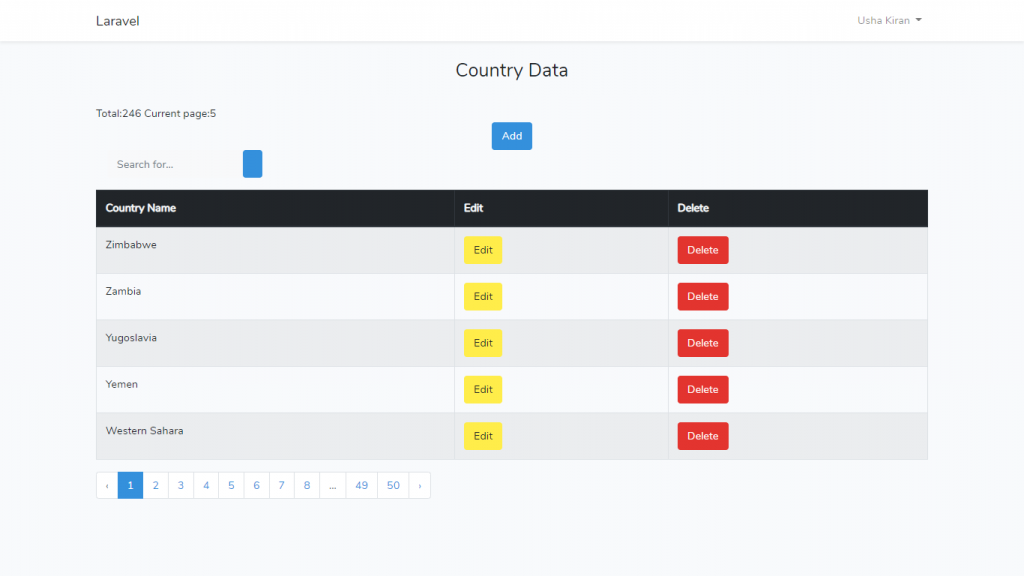
Thanks

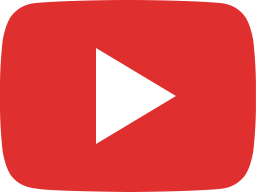

With MotoShare.in, you can book a bike instantly, enjoy doorstep delivery, and ride without worries. Perfect for travelers, professionals, and adventure enthusiasts looking for a seamless mobility solution.