How to print the number in reverse order in PHP?
In this program, you’ll learn to reverse a number using a while loop.
Logic:
- First of all, the remainder of $num divided by 10 is stored in the variable $rem. Now, $rem contains the last digit of $num, i.e. 3.
- $rem is then added to the variable $rev after multiplying it by 10.
- Multiplication by 10 adds a new place in the $rev contains. $rev contain like this 0 * 10 + 3 = 3.
- $num is then divided by 10 so that now it only contains first two digits: 12.
- After second iteration, $rem equals 2, $rev equals 3 * 10 + 2 = 32 and $num = 1.
- After third iteration, $rem equals 2, $rev equals 32 * 10 + 1 = 321 and $num = 0.
- Now, $num will be existed outside the while loop and $rev contains 321.
Now , look at this coding
<?php | |
$message = ''; | |
if (isset($_POST['num'])) { | |
if (!empty($_POST['num'])) { | |
$num = $_POST['num']; | |
$rev=0; | |
$temp =$num; // number is stored for temporary in temp variable | |
while ($temp>1) { | |
$rem = $temp%10; | |
$rev =($rev * 10) + $rem; | |
$temp =($temp / 10); | |
} | |
//echo "<h3> Reverse number of $num is: $rev </h3>"; | |
$message = "Reverse number of $num is: $rev"; | |
}else{ | |
echo "<h4>No number has been ebtered</h4>"; | |
} | |
} | |
?> | |
<!DOCTYPE html> | |
<html> | |
<head> | |
<title>Reverse program</title> | |
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css"> | |
<link rel ="stylesheet" type="text/css" href="css/bootstrap.min.css"> | |
<link href="https://fonts.googleapis.com/css2?family=Balsamiq+Sans&display=swap" rel="stylesheet"> | |
<style> | |
body{ | |
font-size: 25px; | |
font-family: 'Balsamiq Sans', cursive; | |
background-color: #46C7C7; | |
} | |
.container{ | |
width: 50%; | |
margin: 0 auto; | |
border-radius: 5px; | |
background-color: #f2f2f2; | |
padding: 20px; | |
} | |
input[type=text], textarea{ | |
width: 150%; | |
padding: 10px; | |
border: 1px solid #ccc; | |
border-radius: 4px; | |
box-sizing: border-box; | |
resize: vertical; | |
color: #2B3856; | |
} | |
input[type=submit]{ | |
color: white; | |
padding: 15px 20px; | |
border: none; | |
border-radius: 5px; | |
cursor: pointer; | |
float: right; | |
} | |
label{ | |
color: #2B547E; | |
} | |
.col-6{ | |
float: left; | |
width: 25%; | |
margin-top: 6px; | |
align: right; | |
} | |
.row:after{ | |
content: " "; | |
display: table; | |
clear: both; | |
} | |
h2,h3,h4{ | |
text-align: center; | |
} | |
</style> | |
</head> | |
<body> | |
<div class="container"> | |
<?php | |
if (!empty($message)) { | |
?> | |
<div class="row"> | |
<h3> <?php echo $message; ?></h3> | |
</div> | |
<?php | |
} ?> | |
<form action="reverse.php" method="POST"> | |
<div> | |
<label>Enter the number :</label> | |
</div> | |
<div class="row"> | |
<div class="col-6"> | |
<textarea name="num" rows="5" cols="40"></textarea> | |
</div> | |
<div class="col-6"> | |
<input type="submit" class="btn btn-primary btn-lg" name="submit" value="Submit"> | |
</div> | |
</div> | |
</form> | |
</div> | |
</body> | |
</html> |
Input : 12345
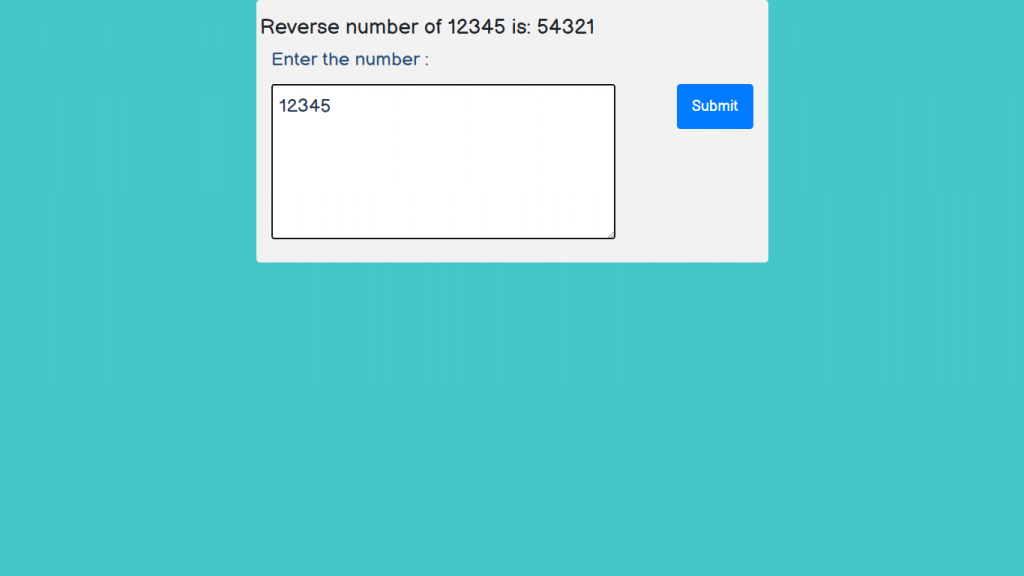
Thanks

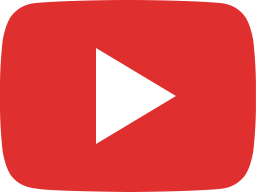
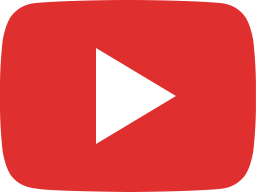

With MotoShare.in, you can book a bike instantly, enjoy doorstep delivery, and ride without worries. Perfect for travelers, professionals, and adventure enthusiasts looking for a seamless mobility solution.