How to Store Countries, States, Cities seed classes into the Database in Laravel PHP?

Step 1. Create a State seeder file. Write down the following command as follows:
$ php artisan make:seeder StatesTableSeeder

Step 2. Create a State model. Write down the following command as follows:
$ php artisan make:model State -m

Step 3. Add columns into Country table.
public function up()
{
Schema::create('states', function (Blueprint $table) {
$table->bigIncrements('state_id');
$table->string('state_name');
$table->integer('country_id');
$table->timestamps();
});
}
Code language: PHP (php)
Step 4. Go to Database/seeds/DatabaseSeeder file. Write down the following command as follows to add State seeder class:
public function run()
{
$this->call(CountriesTableSeeder::class);
$this->call(StatesTableSeeder::class);
}
Code language: PHP (php)
Step 5. Go to Database/seeds/CountriesTableSeeder file.
<?php | |
use App\State; | |
use App\Country; | |
use Illuminate\Database\Seeder; | |
use Illuminate\Support\Facades\DB; | |
class StatesTableSeeder extends Seeder | |
{ | |
/** | |
* Run the database seeds. | |
* | |
* @return void | |
*/ | |
public function run() | |
{ | |
$states = [ | |
[ | |
'state_id' => 1, | |
'country_id'=> 229, | |
'state_name' => 'Alabama' | |
], | |
[ | |
'state_id' => 2, | |
'country_id'=> 229, | |
'state_name' => 'Alaska' | |
], | |
[ | |
'state_id' => 3, | |
'country_id'=>229, | |
'state_name' => 'Arizona' | |
], | |
[ | |
'state_id' => 4, | |
'country_id'=>229, | |
'state_name' => 'Arkansas' | |
], | |
[ | |
'state_id' => 5, | |
'country_id'=>229, | |
'state_name' => 'California' | |
], | |
[ | |
'state_id' => 6, | |
'country_id'=>229, | |
'state_name' => 'Colorado' | |
], | |
[ | |
'state_id' => 7, | |
'country_id'=>229, | |
'state_name' => 'Connecticut' | |
], | |
[ | |
'state_id' => 8, | |
'country_id'=>229, | |
'state_name' => 'Delaware' | |
], | |
[ | |
'state_id' => 9, | |
'country_id'=>229, | |
'state_name' => 'District of Columbia' | |
], | |
[ | |
'state_id' => 10, | |
'country_id'=>229, | |
'state_name' => 'Florida' | |
], | |
[ | |
'state_id' => 11, | |
'country_id'=>229, | |
'state_name' => 'Georgia' | |
], | |
[ | |
'state_id' => 12, | |
'country_id'=>229, | |
'state_name' => 'Hawaii' | |
], | |
[ | |
'state_id' => 13, | |
'country_id'=>229, | |
'state_name' => 'Idaho' | |
], | |
[ | |
'state_id' => 14, | |
'country_id'=>229, | |
'state_name' => 'Illinois' | |
], | |
[ | |
'state_id' => 15, | |
'country_id'=>229, | |
'state_name' => 'Indiana' | |
], | |
[ | |
'state_id' => 16, | |
'country_id'=>229, | |
'state_name' => 'Iowa' | |
], | |
[ | |
'state_id' => 17, | |
'country_id'=>229, | |
'state_name' => 'Kansas' | |
], | |
[ | |
'state_id' => 18, | |
'country_id'=>229, | |
'state_name' => 'Kentucky' | |
], | |
[ | |
'state_id' => 19, | |
'country_id'=>229, | |
'state_name' => 'Louisiana' | |
], | |
[ | |
'state_id' => 20, | |
'country_id'=>229, | |
'state_name' => 'Maine' | |
], | |
[ | |
'state_id' => 21, | |
'country_id'=>229, | |
'state_name' => 'Maryland' | |
], | |
[ | |
'state_id' => 22, | |
'country_id'=>229, | |
'state_name' => 'Massachusetts' | |
], | |
[ | |
'state_id' => 23, | |
'country_id'=>229, | |
'state_name' => 'Michigan' | |
], | |
[ | |
'state_id' => 24, | |
'country_id'=>229, | |
'state_name' => 'Minnesota' | |
], | |
[ | |
'state_id' => 25, | |
'country_id'=>229, | |
'state_name' => 'Mississippi' | |
], | |
[ | |
'state_id' => 26, | |
'country_id'=>229, | |
'state_name' => 'Missouri' | |
], | |
[ | |
'state_id' => 27, | |
'country_id'=>229, | |
'state_name' => 'Montana' | |
], | |
[ | |
'state_id' => 28, | |
'country_id'=>229, | |
'state_name' => 'Nebraska' | |
], | |
[ | |
'state_id' => 29, | |
'country_id'=>95, | |
'state_name' => 'Andhra Pradesh' | |
], | |
[ | |
'state_id' => 30, | |
'country_id'=>95 , | |
'state_name' => 'Arunachal Pradesh ' | |
], | |
[ | |
'state_id' => 31, | |
'country_id'=>95, | |
'state_name' => 'Assam' | |
], | |
[ | |
'state_id' => 32, | |
'country_id'=>95, | |
'state_name' => 'Bihar' | |
], | |
[ | |
'state_id' => 33, | |
'country_id'=>95, | |
'state_name' => 'Chhattisgarh' | |
], | |
[ | |
'state_id' => 34, | |
'country_id'=>95, | |
'state_name' => 'Goa' | |
], | |
[ | |
'state_id' => 35, | |
'country_id'=>95, | |
'state_name' => 'Gujarat' | |
], | |
[ | |
'state_id' => 36, | |
'country_id'=>95, | |
'state_name' => 'Haryana' | |
], | |
[ | |
'state_id' => 37, | |
'country_id'=>95, | |
'state_name' => 'Himachal Pradesh' | |
], | |
[ | |
'state_id' => 38, | |
'country_id'=>95, | |
'state_name' => 'Jharkhand' | |
], | |
[ | |
'state_id' => 39, | |
'country_id'=>95, | |
'state_name' => 'Karnataka' | |
], | |
[ | |
'state_id' => 40, | |
'country_id'=>95, | |
'state_name' => 'Kerala ' | |
], | |
[ | |
'state_id' => 41, | |
'country_id'=>95, | |
'state_name' => 'Madhya Pradesh' | |
], | |
[ | |
'state_id' => 42, | |
'country_id'=>95, | |
'state_name' => 'Maharashtra' | |
], | |
[ | |
'state_id' => 43, | |
'country_id'=>95, | |
'state_name' => 'Manipur' | |
], | |
[ | |
'state_id' => 44, | |
'country_id'=>95, | |
'state_name' => 'Meghalaya' | |
], | |
[ | |
'state_id' => 45, | |
'country_id'=>95, | |
'state_name' => 'Mizoram' | |
], | |
[ | |
'state_id' => 46, | |
'country_id'=>95, | |
'state_name' => 'Nagaland' | |
], | |
[ | |
'state_id' => 47, | |
'country_id'=>95, | |
'state_name' => 'Odisha' | |
], | |
[ | |
'state_id' => 48, | |
'country_id'=>95, | |
'state_name' => 'Punjab' | |
], | |
[ | |
'state_id' => 49, | |
'country_id'=>95, | |
'state_name' => 'Rajasthan' | |
], | |
[ | |
'state_id' => 50, | |
'country_id'=>95, | |
'state_name' => 'Sikkim' | |
], | |
[ | |
'state_id' => 51, | |
'country_id'=>95, | |
'state_name' => 'Tamil Nadu' | |
], | |
[ | |
'state_id' => 52, | |
'country_id'=>95, | |
'state_name' => 'Telangana ' | |
], | |
[ | |
'state_id' => 53, | |
'country_id'=>95, | |
'state_name' => 'Tripura ' | |
], | |
[ | |
'state_id' => 54, | |
'country_id'=>95, | |
'state_name' => 'Uttar Pradesh' | |
], | |
[ | |
'state_id' => 55, | |
'country_id'=>95, | |
'state_name' => 'Uttarakhand' | |
], | |
[ | |
'state_id' => 56, | |
'country_id'=>95, | |
'state_name' => 'West Bengal ' | |
], | |
[ | |
'state_id' => 57, | |
'country_id'=>95, | |
'state_name' => ' Andaman and Nicobar Islands ' | |
], | |
[ | |
'state_id' => 58, | |
'country_id'=>95, | |
'state_name' => 'Chandigarh' | |
], | |
[ | |
'state_id' => 59, | |
'country_id'=>95, | |
'state_name' => 'Dadra and Nagar Haveli and Daman and Diu (DNHDD)' | |
], | |
[ | |
'state_id' => 60, | |
'country_id'=>95, | |
'state_name' => 'Delhi' | |
], | |
[ | |
'state_id' => 61, | |
'country_id'=>95, | |
'state_name' => 'Jammu and Kashmir ' | |
], | |
[ | |
'state_id' => 62, | |
'country_id'=>95, | |
'state_name' => 'Ladakh ' | |
], | |
[ | |
'state_id' => 63, | |
'country_id'=>95, | |
'state_name' => 'Lakshadweep' | |
], | |
[ | |
'state_id' => 64, | |
'country_id'=>95, | |
'state_name' => 'Puducherry' | |
], | |
]; | |
State::insert($states); | |
} | |
} |
Step 6. Migrate the tables into the MySQL database.
$ php artisan migrate
Step 7. Run the seeder class file individually.
$ php artisan db:seed --class=StatesTableSeeder
Code language: JavaScript (javascript)
Step 8. Go to app\http\Controllers\MainController.php file. Define funcitions of Country and State.
<?php | |
namespace App\Http\Controllers; | |
use Illuminate\Http\Request; | |
use App\Country; | |
use App\State; | |
use App\City; | |
class MainController extends Controller | |
{ | |
public function index(){ | |
$countries = Country::all()->pluck('country_name','country_id'); | |
return view('welcome',compact('countries')); | |
} | |
public function getStates($id){ | |
$states= State::where('country_id',$id)->get(); | |
return response()->json(['states' => $states]); | |
} | |
} |
Step 9. Then, go to routes/web.php file and define all these routes.
Route::get('/getStates/{id}','MainController@getStates');
Code language: JavaScript (javascript)

Thanks

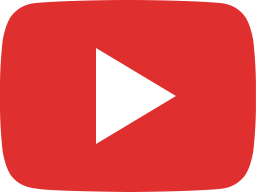

With MotoShare.in, you can book a bike instantly, enjoy doorstep delivery, and ride without worries. Perfect for travelers, professionals, and adventure enthusiasts looking for a seamless mobility solution.