How to Store Countries, States, Cities seed classes into the Database in Laravel PHP
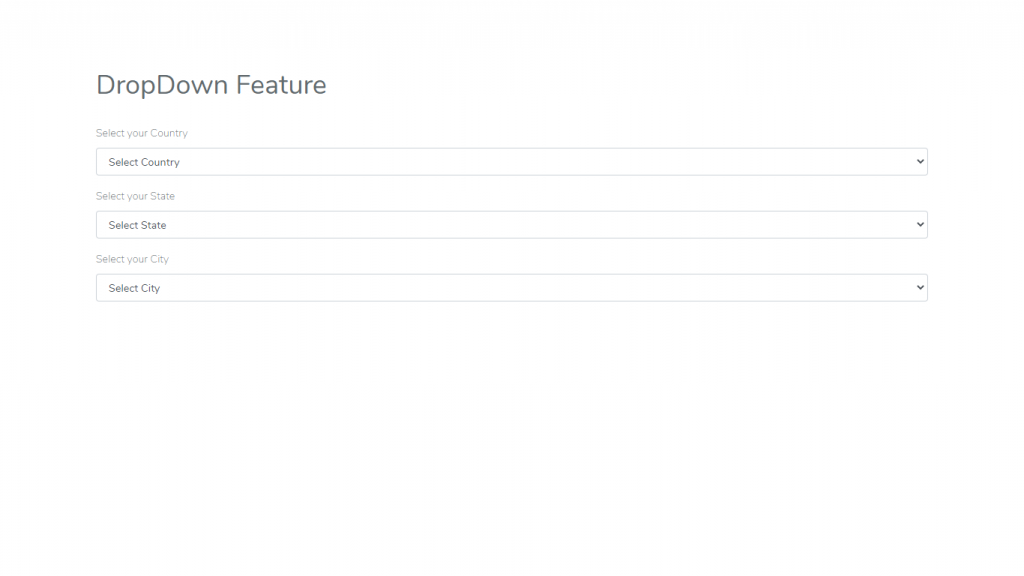
Step 1. Create a City seeder file. Write down the following command as follows:
$ php artisan make:seeder CitiesTableSeeder
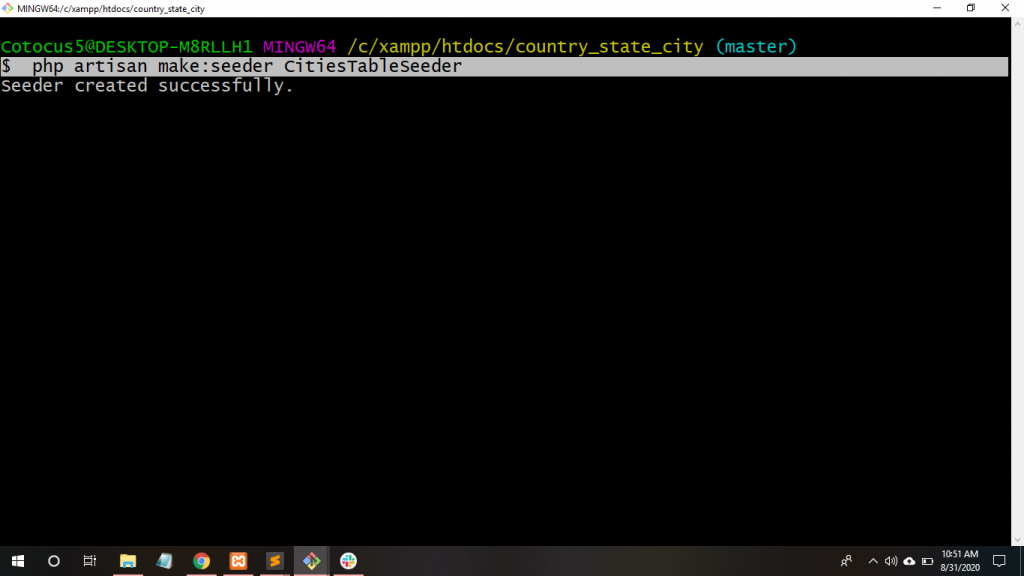
Step 2. Create a City model. Write down the following command as follows:
$ php artisan make:model City -m
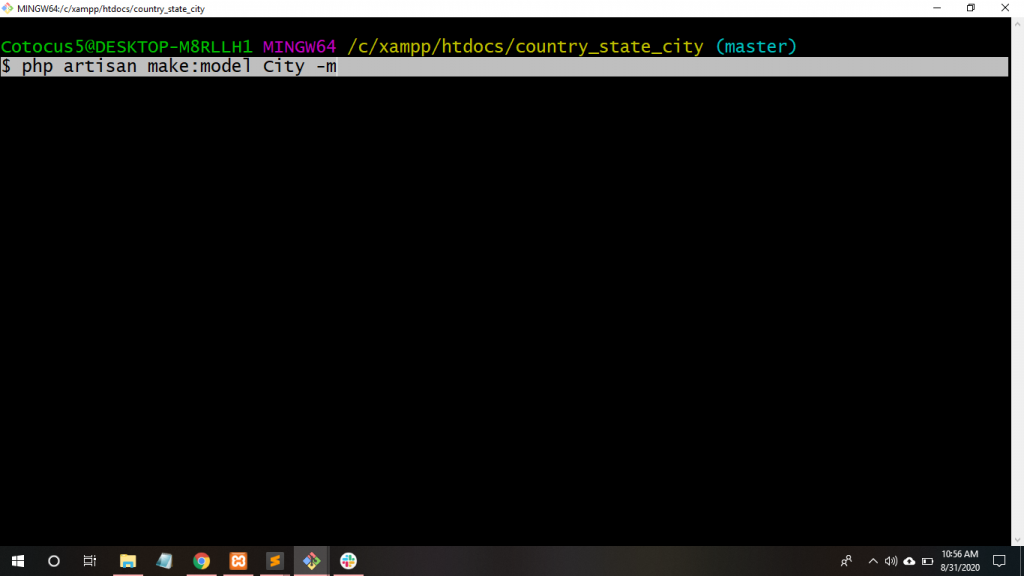
Step 3. Add columns into Country table.
public function up()
{
Schema::create('cities', function (Blueprint $table) {
$table->bigIncrements('city_id');
$table->string('city_name');
$table->integer('state_id');
$table->timestamps();
});
}
Step 4. Go to Database/seeds/DatabaseSeeder file. Write down the following command as follows to add City seeder class:
$this->call(CitiesTableSeeder::class);
public function run()
{
$this->call(CountriesTableSeeder::class);
$this->call(StatesTableSeeder::class);
$this->call(CitiesTableSeeder::class);
}
Step 5. Go to Database/seeds/CitiesTableSeeder file.
<?php | |
use App\City; | |
use App\State; | |
use App\Country; | |
use Illuminate\Database\Seeder; | |
use Illuminate\Support\Facades\DB; | |
class CitiesTableSeeder extends Seeder | |
{ | |
/** | |
* Run the database seeds. | |
* | |
* @return void | |
*/ | |
public function run() | |
{ | |
$cities = [ | |
[ | |
'city_id' => 1, | |
'state_id'=> 16, | |
'city_name' => 'Amlabad' | |
], | |
[ | |
'city_id' => 2, | |
'state_id'=> 16, | |
'city_name' => 'Bandh Dih' | |
], | |
[ | |
'city_id' => 3, | |
'state_id'=> 16, | |
'city_name' => 'Bandhgora' | |
], | |
[ | |
'city_id' => 4, | |
'state_id'=> 16, | |
'city_name' => 'Bhojudih' | |
], | |
[ | |
'city_id' => 5, | |
'state_id'=> 16, | |
'city_name' => 'Bokaro Steel City' | |
], | |
[ | |
'city_id' => 16, | |
'state_id'=> 16, | |
'city_name' => 'Chandrapura' | |
], | |
[ | |
'city_id' => 7, | |
'state_id'=> 16, | |
'city_name' => 'Chas' | |
], | |
[ | |
'city_id' => 8, | |
'state_id'=> 16, | |
'city_name' => 'Chira Chas' | |
], | |
[ | |
'city_id' => 9, | |
'state_id'=> 16, | |
'city_name' => 'Dugda' | |
], | |
[ | |
'city_id' => 10, | |
'state_id'=> 16, | |
'city_name' => 'Dungaditand' | |
], | |
[ | |
'city_id' => 11, | |
'state_id'=> 16, | |
'city_name' => 'Gomia' | |
], | |
[ | |
'city_id' => 12, | |
'state_id'=> 16, | |
'city_name' => 'Jaridih Bazar' | |
], | |
[ | |
'city_id' => 13, | |
'state_id'=> 16, | |
'city_name' => 'Jena' | |
], | |
[ | |
'city_id' => 14, | |
'state_id'=> 16, | |
'city_name' => 'Kurpania' | |
], | |
[ | |
'city_id' => 15, | |
'state_id'=> 16, | |
'city_name' => 'Lalpania' | |
], | |
[ | |
'city_id' => 101, | |
'state_id'=> 16, | |
'city_name' => 'Phusro' | |
], | |
[ | |
'city_id' => 17, | |
'state_id'=> 16, | |
'city_name' => 'Sijhua' | |
], | |
[ | |
'city_id' => 18, | |
'state_id'=> 16, | |
'city_name' => 'Tenu' | |
], | |
[ | |
'city_id' => 19, | |
'state_id'=> 1, | |
'city_name' => 'bombuflat' | |
], | |
[ | |
'city_id' => 20, | |
'state_id'=> 1, | |
'city_name' => 'Garacharma' | |
], | |
[ | |
'city_id' => 21, | |
'state_id'=> 1, | |
'city_name' => 'Port Blair' | |
], | |
[ | |
'city_id' => 22, | |
'state_id'=> 1, | |
'city_name' => 'Rangat' | |
], | |
[ | |
'city_id' => 23, | |
'state_id'=> 2, | |
'city_name' => 'Addanki' | |
], | |
[ | |
'city_id' => 24, | |
'state_id'=> 2, | |
'city_name' => 'Adivivaram' | |
], | |
[ | |
'city_id' => 25, | |
'state_id'=> 2, | |
'city_name' => 'Adoni' | |
], | |
[ | |
'city_id' => 26, | |
'state_id'=> 2, | |
'city_name' => 'Aganampudi' | |
], | |
[ | |
'city_id' => 27, | |
'state_id'=> 2, | |
'city_name' => 'Ajjaram' | |
], | |
[ | |
'city_id' => 28, | |
'state_id'=> 2, | |
'city_name' => 'Akividu' | |
], | |
[ | |
'city_id' => 29, | |
'state_id'=> 2, | |
'city_name' => 'Akkarampalle' | |
], | |
[ | |
'city_id' => 30, | |
'state_id'=> 2, | |
'city_name' => 'Akkayapalle' | |
], | |
[ | |
'city_id' => 31, | |
'state_id'=> 2, | |
'city_name' => 'Akkireddipalem' | |
], | |
[ | |
'city_id' =>32, | |
'state_id'=> 2, | |
'city_name' => 'Alampur' | |
], | |
[ | |
'city_id' => 33, | |
'state_id'=> 2, | |
'city_name' => 'Amalapuram' | |
], | |
]; | |
City::insert($cities); | |
} | |
} |
Step 6. Migrate the tables into the MySQL database.
$ php artisan migrate
Step 7. Run the seeder class file individually.
$ php artisan db:seed --class=CitiesTableSeeder
Step 8. Go to app\http\Controllers\MainController.php file. Define funcitions of City.
public function getCities($id){
$cities= City::where('state_id',$id)->get();
return response()->json(['cities' => $cities]);
}
Step 9. Then, go to routes/web.php file and define all these routes.
Route::get('/getCities/{id}','MainController@getCities');
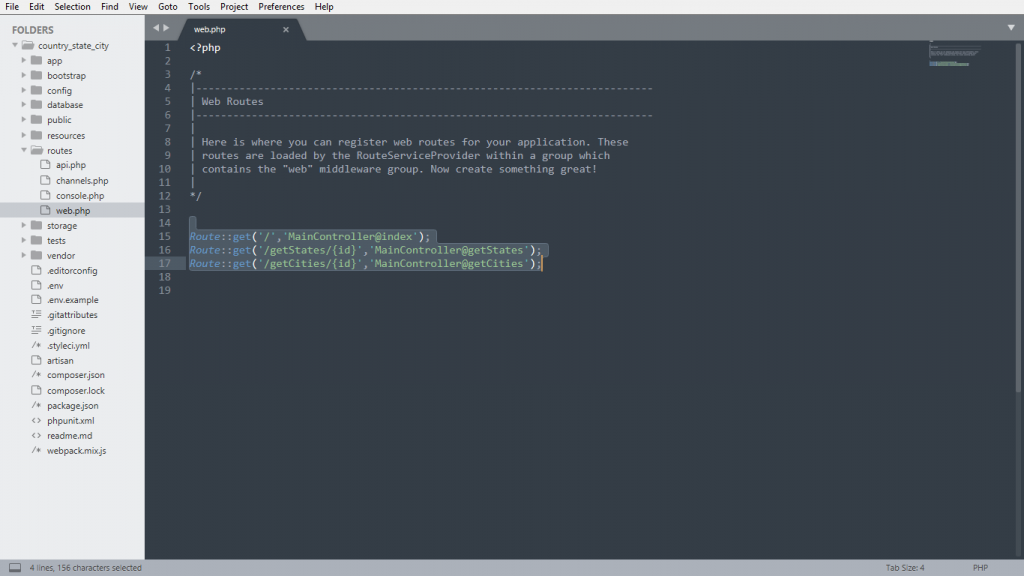
Step 10. Go to resources/views/welcome.blade.php file
<div class="container"> | |
<br/><br/><br/> | |
<h1>DropDown Feature</h1> | |
<br/> | |
<div class="form-group"> | |
<label for="country">Select your Country</label> | |
<select name="country_id" id="country" class="form-control"> | |
<option value="">Select Country</option> | |
@foreach($countries as $key => $value) | |
<option value="{{$key}}">{{$value}}</option> | |
@endforeach | |
</select> | |
</div> | |
<div class="form-group"> | |
<label for="state">Select your State</label> | |
<select name="state_id" id="state" class="form-control"> | |
<option value="">Select State</option> | |
</select> | |
</div> | |
<div class="form-group"> | |
<label for="city">Select your City</label> | |
<select name="city_id" id="city" class="form-control"> | |
<option value="">Select City</option> | |
</select> | |
</div> | |
</div> | |
<script src="{{asset('js/jquery.js')}}"></script> | |
<script> | |
$(document).ready(function(){ | |
$('#country').on('change',function(){ | |
var country_id= $(this).val(); | |
if (country_id) { | |
$.ajax({ | |
url: "{{url('/getStates')}}/"+country_id, | |
type: "GET", | |
dataType: "json", | |
success: function(html){ | |
console.log(html.states); | |
$('select[name="state_id"]').empty(); | |
$.each(html.states,function(i, states){ | |
console.log(states.state_id); | |
$('select[name="state_id"]').append('<option value="'+states.state_id+'">'+states.state_name+'</option>'); | |
}); | |
} | |
}); | |
}else { | |
$('select[name="state_id"]').empty(); | |
} | |
}); | |
$('#state').on('change',function(){ | |
var state_id= $(this).val(); | |
if (state_id) { | |
$.ajax({ | |
url: "{{url('/getCities')}}/"+state_id, | |
type: "GET", | |
dataType: "json", | |
success: function(html){ | |
console.log(html.cities); | |
$('select[name="city_id"]').empty(); | |
$.each(html.cities,function(i, cities){ | |
console.log(cities.city_id); | |
$('select[name="city_id"]').append('<option value="'+cities.city_id+'">'+cities.city_name+'</option>'); | |
}); | |
} | |
}); | |
}else { | |
$('select[name="city_id"]').empty(); | |
} | |
}); | |
}); | |
</script> | |
</script> |
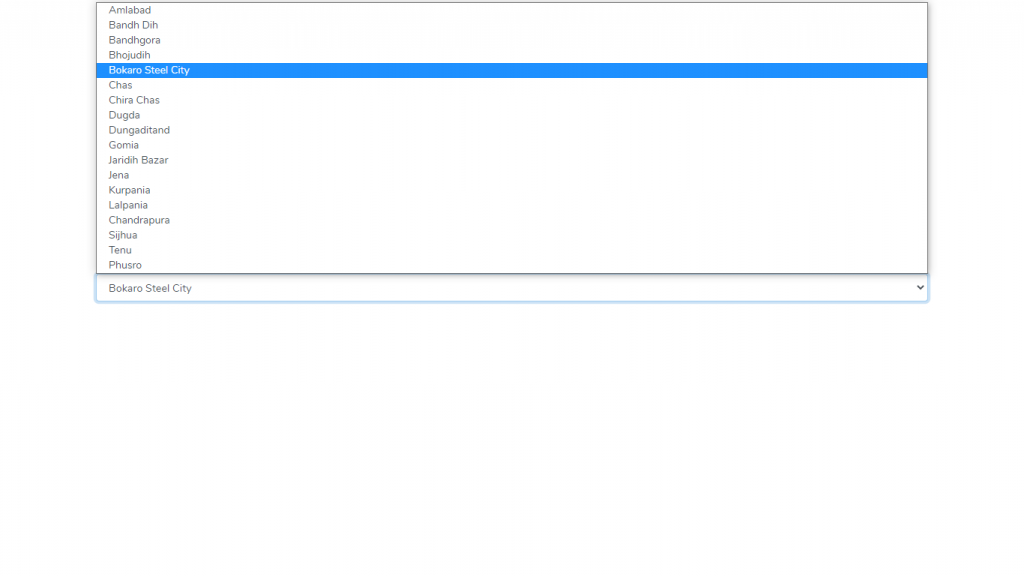
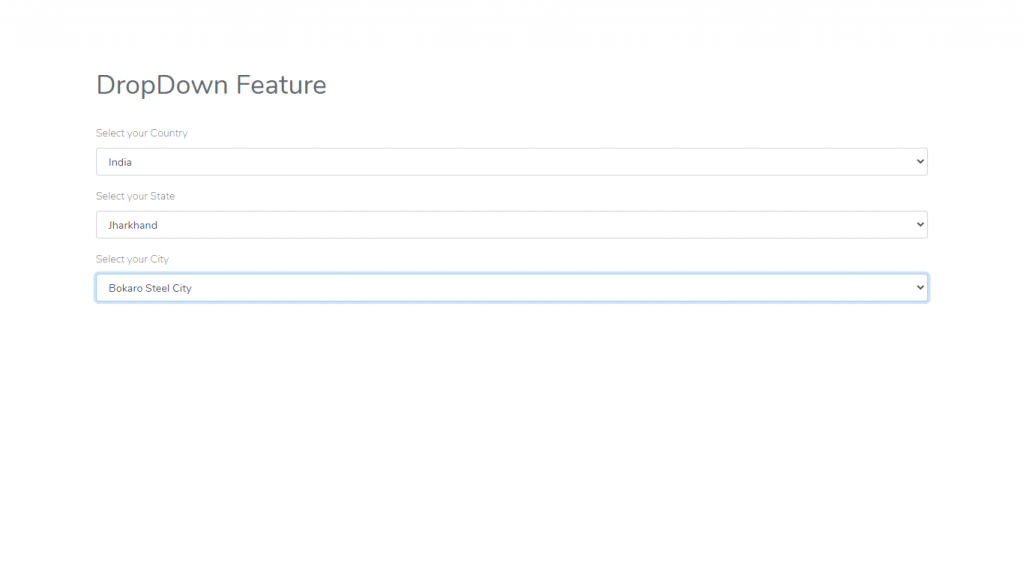
Thanks

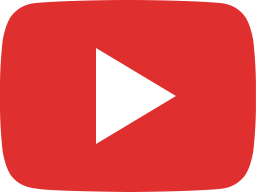

With MotoShare.in, you can book a bike instantly, enjoy doorstep delivery, and ride without worries. Perfect for travelers, professionals, and adventure enthusiasts looking for a seamless mobility solution.