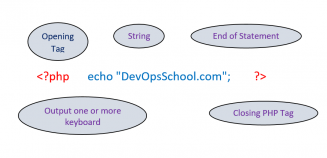
What is PHP?
PHP: Hypertext Preprocessor(Recursive Acronym)
Server Side Programming/Scripting Language
Can be embedded directly within HTML (<?php ?>)
Files use a “.php” file extension.

If… statement:-
<?php | |
$a = 5; | |
if ($a >1) | |
{ | |
echo "this is if.. statement"; //if this is true then print if wrong then blank | |
} | |
?> |
if… and nested if :-
<?php | |
if(10<12) | |
{ | |
echo "this is right <br>"; //when true total echo print and when wrong then wrong statement print one or more | |
if (22>21) | |
{ | |
echo "this is too right<br>"; | |
} | |
if (45<40) | |
{ | |
echo "this is false <br>"; | |
} | |
if(44>30) | |
{ | |
echo "this is wrong <br>"; | |
} | |
} | |
echo "this is end"; | |
?> |
if…else Statement:-
<?php | |
$a = 4; //before check condition if condition is true then print if wrong the print false | |
if ($a < 1) { | |
echo "This is True"; | |
} else { | |
echo "This is False <br>"; | |
} | |
//second example | |
$a = 4; | |
if ($a < 6) { | |
echo "This is True!"; | |
} else { | |
echo "This is False"; | |
} | |
?> |
if…elseif…else Statement:-
<?php | |
if(5>7) | |
{ | |
echo "this is wrong"; //check statement if right then print if wrong then next check | |
} | |
elseif(8>9) | |
{ | |
echo "this is again wrong"; | |
} | |
elseif(9>12) | |
{ | |
echo "this is too wrong"; | |
} | |
else | |
echo "this is last"; | |
echo "<br>"; | |
//second example | |
$price = 20; | |
if ($price < 10) | |
{ | |
echo "this is not right "; | |
} | |
elseif ($price < 20) | |
{ | |
echo "this is again not right"; | |
} | |
elseif ($price < 20) | |
{ | |
echo "this is too not right"; | |
} | |
else | |
echo "this is end"; | |
?> |
While Loop:-
<?php | |
//while loop | |
$value = 1; | |
while($value <= 5) //before check if conditon is true then executed if wrong then exit | |
{ | |
echo "this is while loop : $value <br>"; | |
++$value; | |
} | |
?> |
Nested While loop:-
<?php | |
#nested while loop | |
$a = 3; | |
while ($a <= 4) //before check if conditon is true then executed internal parts with check internal while loop conditions | |
//if wrong then exit | |
{ | |
echo "this is example $a <br>"; | |
++$a; | |
$b = 4; | |
while ($b <= 5) | |
{ | |
echo "val $b <br>"; | |
++$b; | |
} | |
} | |
?> |
Do While loop:-
<?php | |
$a = 5; | |
do //before executed after condition check | |
{ | |
echo "this is do while loop $a <br>"; | |
$a++; | |
} while ($a <= 6); | |
//second example | |
$b = 3; | |
do | |
{ | |
echo "this is do... while loop $a <br>"; | |
++$b; | |
} while ($b <= 5); | |
?> |
Nested Do While loop:-
<?php | |
$a = 4; //before executed after condition check but one or more | |
do | |
{ | |
echo "this is first $a <br>"; | |
$a++; | |
$b = 5; | |
do | |
{ | |
echo "this is second $b <br>"; //first check | |
++$b; | |
} while ($b <= 7); | |
} while ($a<= 6); //second check | |
?> |
For loop :-
<?php | |
for ($a = 2; $a< 5; ++$a) // before check if conditon is true then print and check next if condition is false then exit | |
{ | |
echo "this is for loop <br>"; | |
} | |
//second example | |
for ($b = 3; $b< 6; ++$b) | |
{ | |
echo "for <br>"; | |
for ($c = 4; $c <7; ++$c) | |
{ | |
echo "nested for <br>"; | |
} | |
} | |
?> |
Nested for loop :-
<?php | |
//Nested for loop | |
for ($b = 3; $b< 6; ++$b) // before check if conditon is true then print and check next if condition is false then exit | |
{ | |
echo "for <br>"; | |
for ($c = 4; $c <7; ++$c) | |
{ | |
echo "nested for <br>"; | |
} | |
} | |
?> |

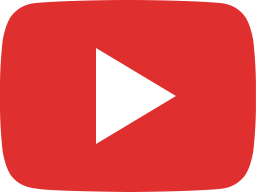
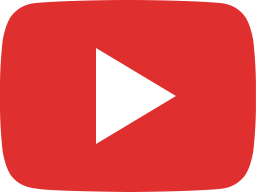

I’m a DevOps/SRE/DevSecOps/Cloud Expert passionate about sharing knowledge and experiences. I am working at Cotocus. I blog tech insights at DevOps School, travel stories at Holiday Landmark, stock market tips at Stocks Mantra, health and fitness guidance at My Medic Plus, product reviews at I reviewed , and SEO strategies at Wizbrand.
Please find my social handles as below;
Rajesh Kumar Personal Website
Rajesh Kumar at YOUTUBE
Rajesh Kumar at INSTAGRAM
Rajesh Kumar at X
Rajesh Kumar at FACEBOOK
Rajesh Kumar at LINKEDIN
Rajesh Kumar at PINTEREST
Rajesh Kumar at QUORA
Rajesh Kumar at WIZBRAND