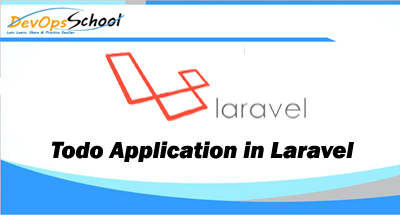
1.Create Project in Laravel
Composer create-project laravel/laravel todolist
2.Create CRUD Controller
php artisan make:controller TodosController –resource
3.Route setup of CRUD Controller
routes/web.php
[code language=”sql”]
Route::get(‘/’,’TodosController@index’);
Route::resource(‘todo’, ‘TodosController’);
[/code]
4.Create Database todolist
localhost/phpmyadmin
create database todolist
5.Create Model Todo
php artisan make:model Todo -m
6.Create Todo Table Structure
database/migrations/_create_todos_table.php
database/migrations/_create_todos_table.php
[code language=”sql”]
public function up()
{
Schema::create(‘todos’, function (Blueprint $table){
$table->increments(‘id’);
$table->string(‘text’);
$table->mediumText(‘body’);
$table->string(‘due’);
$table->timestamps();
});
}
[/code]
7.Database Configuration
.env
[code language=”sql”]
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=todolist
DB_USERNAME=root
DB_PASSWORD=
[/code]
8.Configure Default String Length
app/Providers/AppServiceProvider.php
[code language=”sql”]
use Illuminate\Support\ServiceProvider;
use Illuminate\Support\Facades\Schema;
public function boot()
{
Schema::defaultStringLength(191);
}
[/code]
9.Migrate with Database
php artisan migrate
10.Data Insert By Command Line
php artisan tinker
[code language=”sql”]</p><p>$todo = new App\Todo();<br> $todo->text=’Todo One’;<br> $todo->body = ‘This is my first todo’;<br> $todo->due = ‘Monday May 7′;<br> $todo->save();</p><p>$todo = new App\Todo();<br> $todo->text=’Todo Two’;<br> $todo->body = ‘This is my second todo’;<br> $todo->due = ‘Tuesday May 8’;<br> $todo->save();</p><p>[/code]
11.Select All Data from Command Line
[code language=”sql”] </p><p>App\Todo::all();</p><cite> <br>[/code]
12.Select Specific Data from Command Line
[code language=”sql”]
>>> App\Todo::find(1);
[/code]
13.Display All Data in index function
app/Http/Controllers/TodosController.php
[code language=”sql”]
use Illuminate\Http\Request;
use App\Todo;
class TodosController extends Controller
{
public function index()
{
// $todos = Todo::all();
$todos = Todo::orderBy(‘created_at’, ‘desc’)->get();
return view(‘todos.index’)->with(‘todos’, $todos);
}
}
[/code]
14.Create index.blade.php file for display index function
resources/views/todos/index.blade.php
[code language=”sql”]
@extends(‘layouts.app’)
@section(‘content’)
<h1>Todos</h1>
@if(count($todos) > 0)
@foreach($todos as $todo)
<div class="card">
<h3><a href="todo/{{$todo->id}}">{{$todo->text}}</a></h3>
<p class="text-danger">{{$todo->due}}</p>
</div>
@endforeach
@endif
@endsection
[/code]
15.create app.blade.php for include css and container
resources/views/layouts/app.blade.php
[code language=”sql”]
<title>TodoList</title>
<link rel="stylesheet" href="/css/app.css">
<div class="container">
@yield(‘content’)
</div>
<footer id ="footer" class="footer">
<p>copyright © 2017 TodoList</p>
</footer>
[/code]
16.Display The Specific Data in show function
app/Http/Controllers/TodosController.php
[code language=”sql”]
use Illuminate\Http\Request;
use App\Todo;
class TodosController extends Controller
{
public function show($id)
{
$todos = Todo::find($id);
return view(‘todos.index’)->with(‘todos’, $todos);
}
}
[/code]
17.Create show.blade.php file for display show function
resources/views/todos/show.blade.php
[code language=”sql”]
@extends(‘layouts.app’)
@section(‘content’)
<a href="/" class="btn btn-default">Go Back</a>
<h1>{{$todo->text}}</h1>
@endsection
[/code]

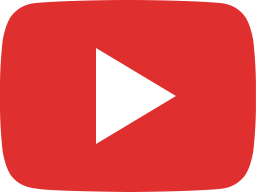

I’m a DevOps/SRE/DevSecOps/Cloud Expert passionate about sharing knowledge and experiences. I am working at Cotocus. I blog tech insights at DevOps School, travel stories at Holiday Landmark, stock market tips at Stocks Mantra, health and fitness guidance at My Medic Plus, product reviews at I reviewed , and SEO strategies at Wizbrand.
Please find my social handles as below;
Rajesh Kumar Personal Website
Rajesh Kumar at YOUTUBE
Rajesh Kumar at INSTAGRAM
Rajesh Kumar at X
Rajesh Kumar at FACEBOOK
Rajesh Kumar at LINKEDIN
Rajesh Kumar at PINTEREST
Rajesh Kumar at QUORA
Rajesh Kumar at WIZBRAND